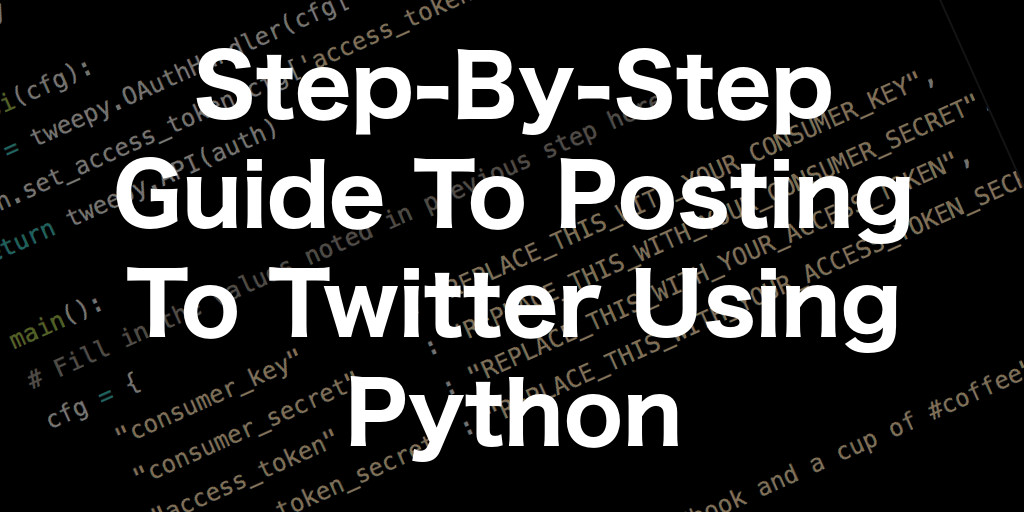
Four Simple Steps To Post To Twitter Using Python
NOTE:
Don't miss the part 2 of this blog post: Posting Photos to Twitter with Python
Automating Twitter can be an easy coding exercise once you have everything set up, but figuring out all the steps that Twitter puts you through to generate your access keys and tokens can be a challenge. I put these four steps together to make it easier to walk to the process, hopefully it's useful for someone else out there.
- Create An Application On app.twitter.com
- Install Python Dependencies
- Write The Python Code
- Post Your First Tweet
TLDR;
If you just want the code, you can grab the code repo for this tutorial here: https://github.com/mattccrampton/post_to_twitter_using_python
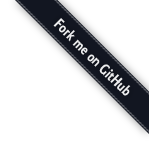
Step #1 - Create An Application on app.twitter.com
First head over to https://apps.twitter.com/, sign in to your twitter account and then click on the "Create New App" button.
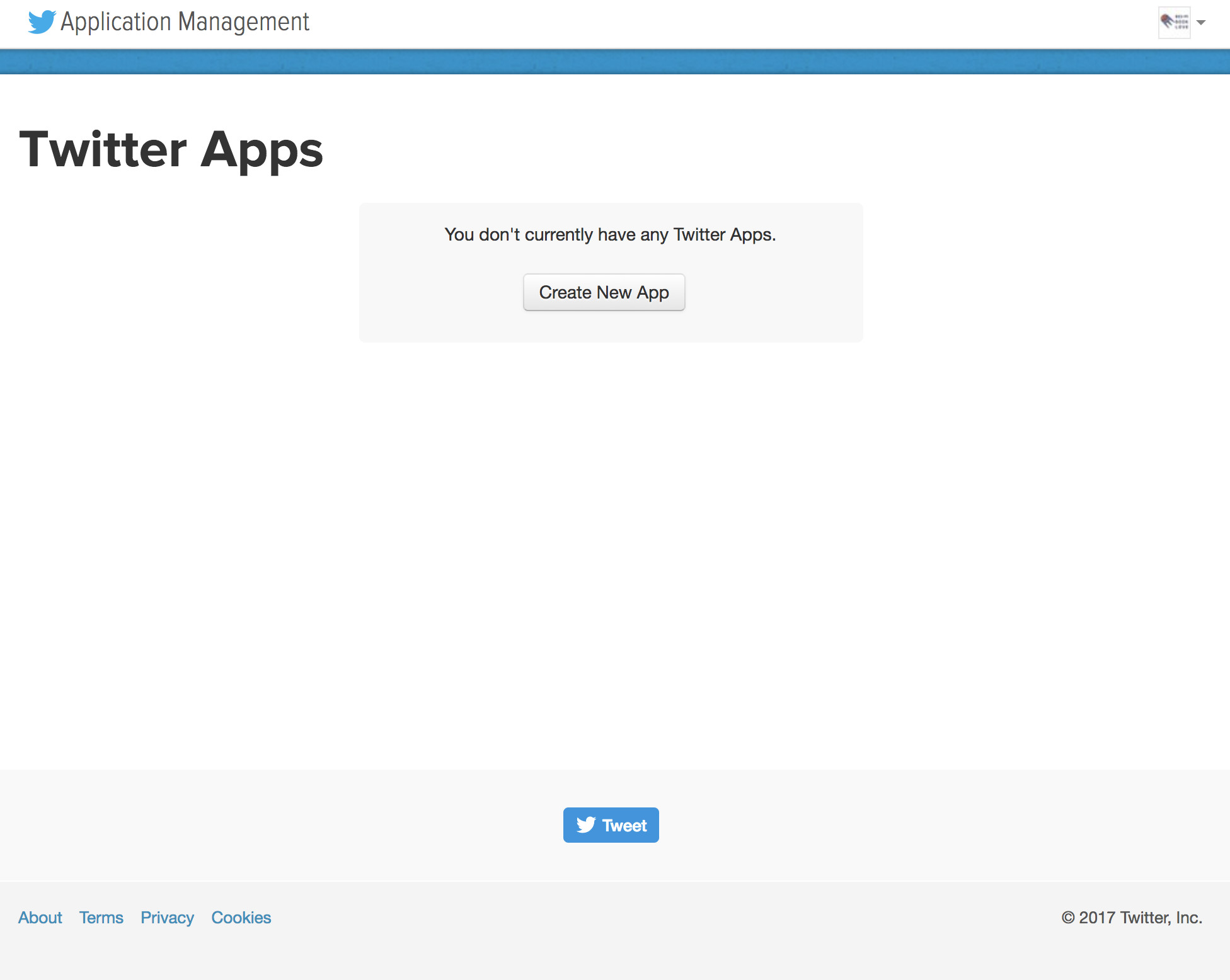
Enter your application's information. For the purposes of this demo I'm creating an application to post to the @scifibooklove twitter acount, so I'm entering information relevant to that website.
Note: Leave the Callback URL field blank.
Once you're done, click "Create your Twitter application".
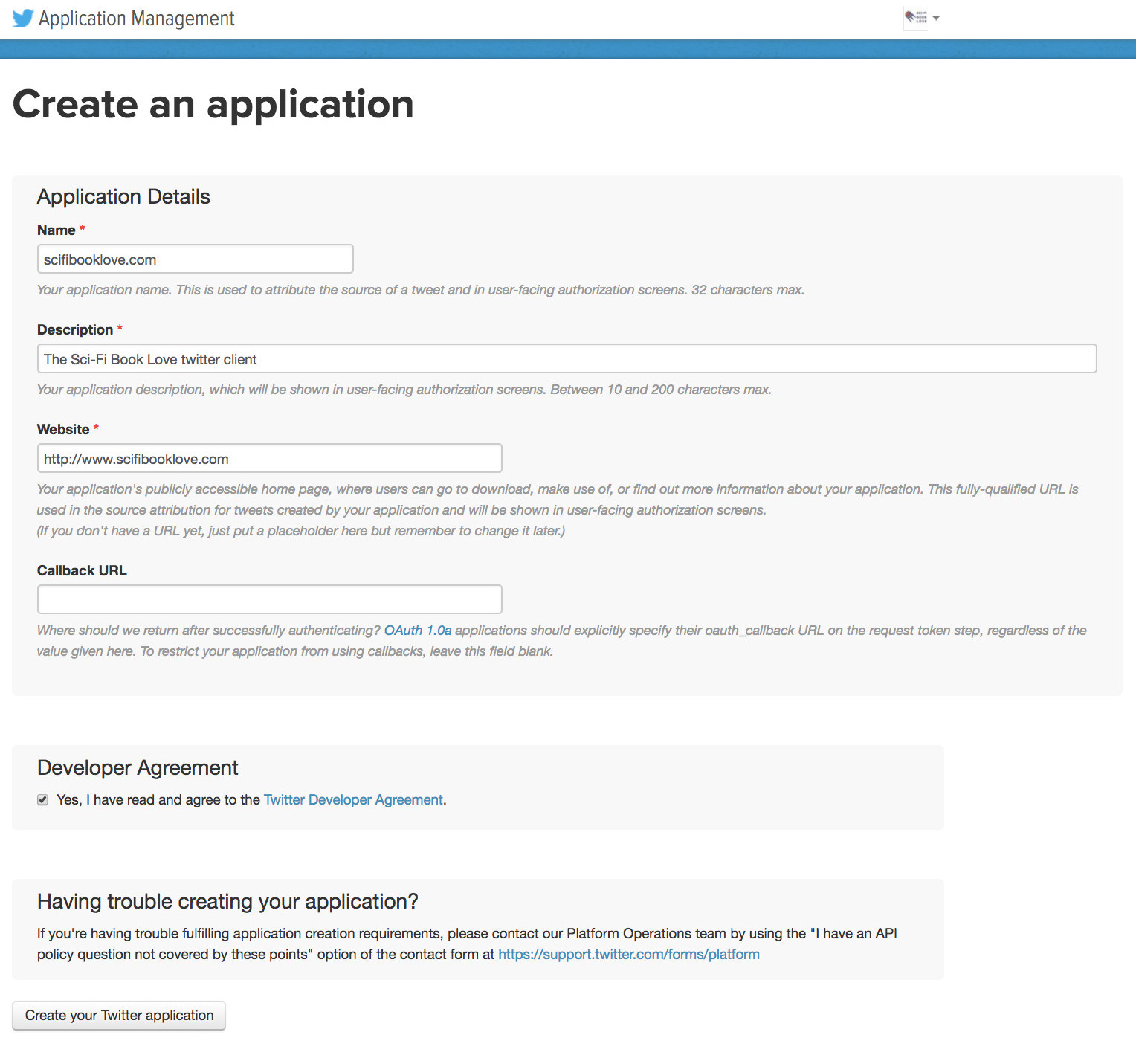
You'll be taken to its detail page with some standard information about the app you've created. Much of this information will be relevant if you decide in the future to build twitter authentication into your web or mobile app or to authenticate on behalf of other users.
But for our simple example we won't be needing much of the data on this page.
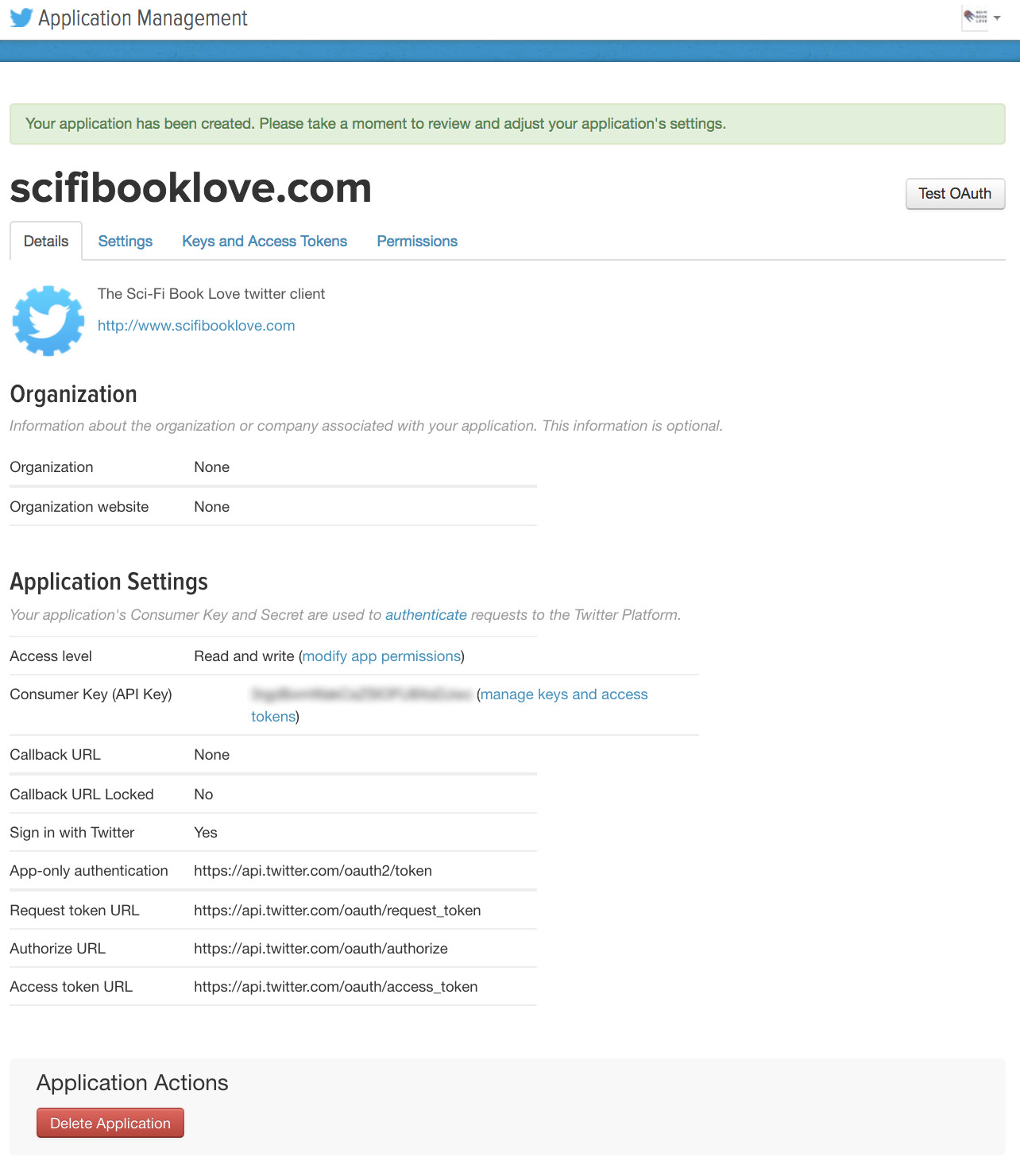
Click over to the "Keys and Access Tokens" tab. This page shows your "Consumer Key" and "Consumer Secret" which are two of the four twitter-generated strings we're going to need to pass into the python code we're going to write.
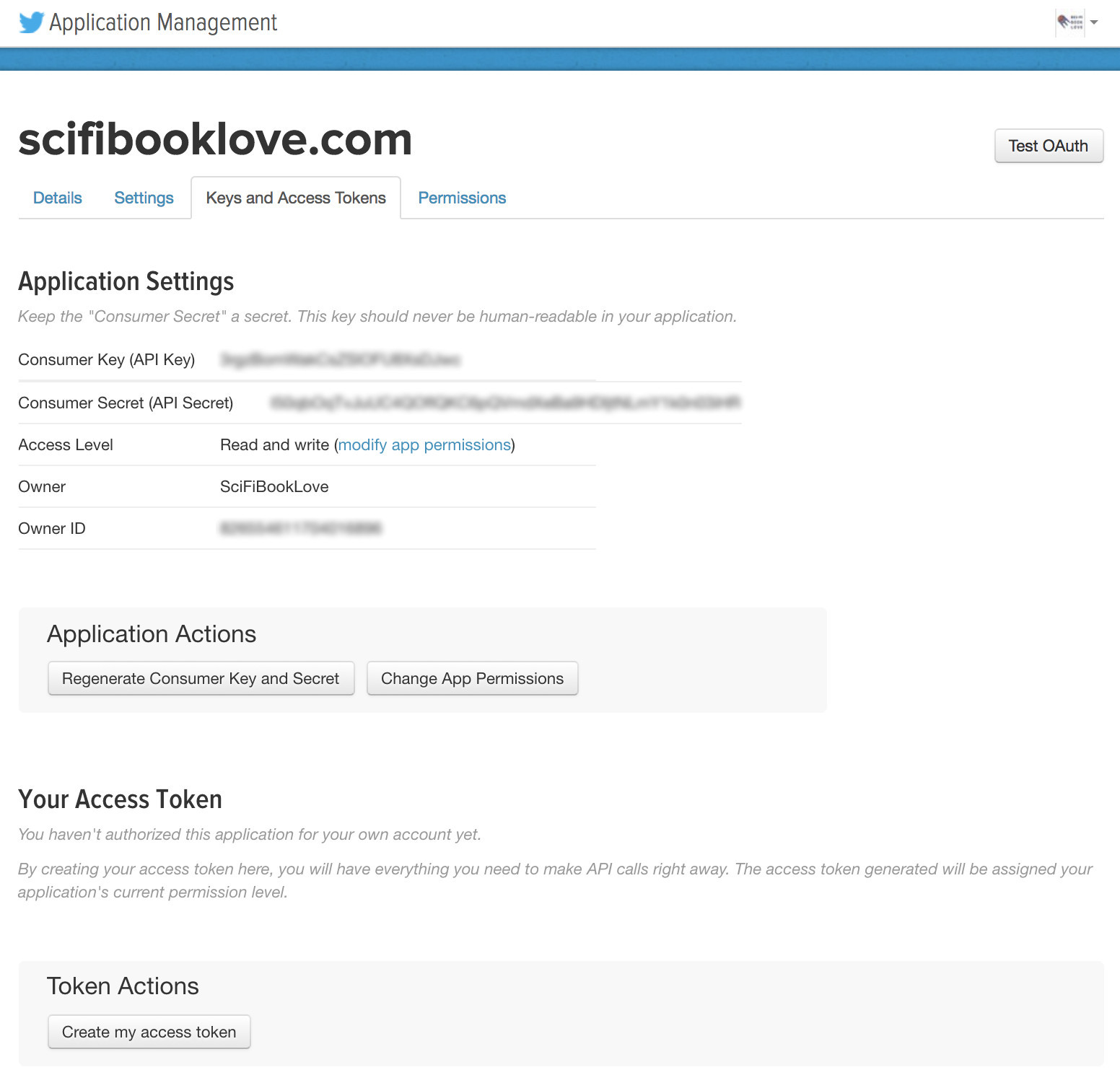
Now click on the "Create my access token" button.
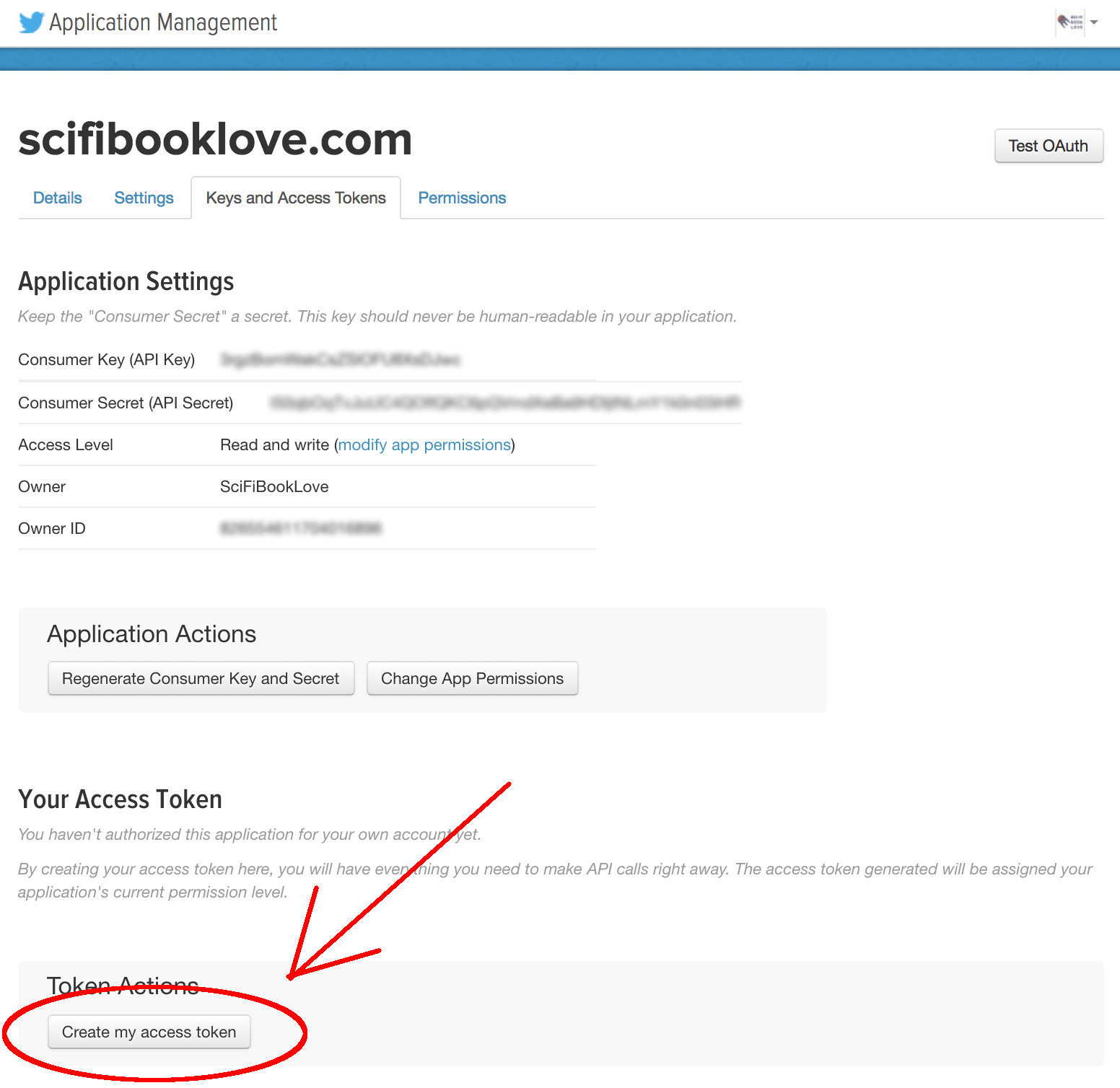
This generates access tokens that allow your new application to access your twitter account (and post on your behalf).
Copy these down along with your Consumer Key and Consumer Secret and save them for your python code (coming up)
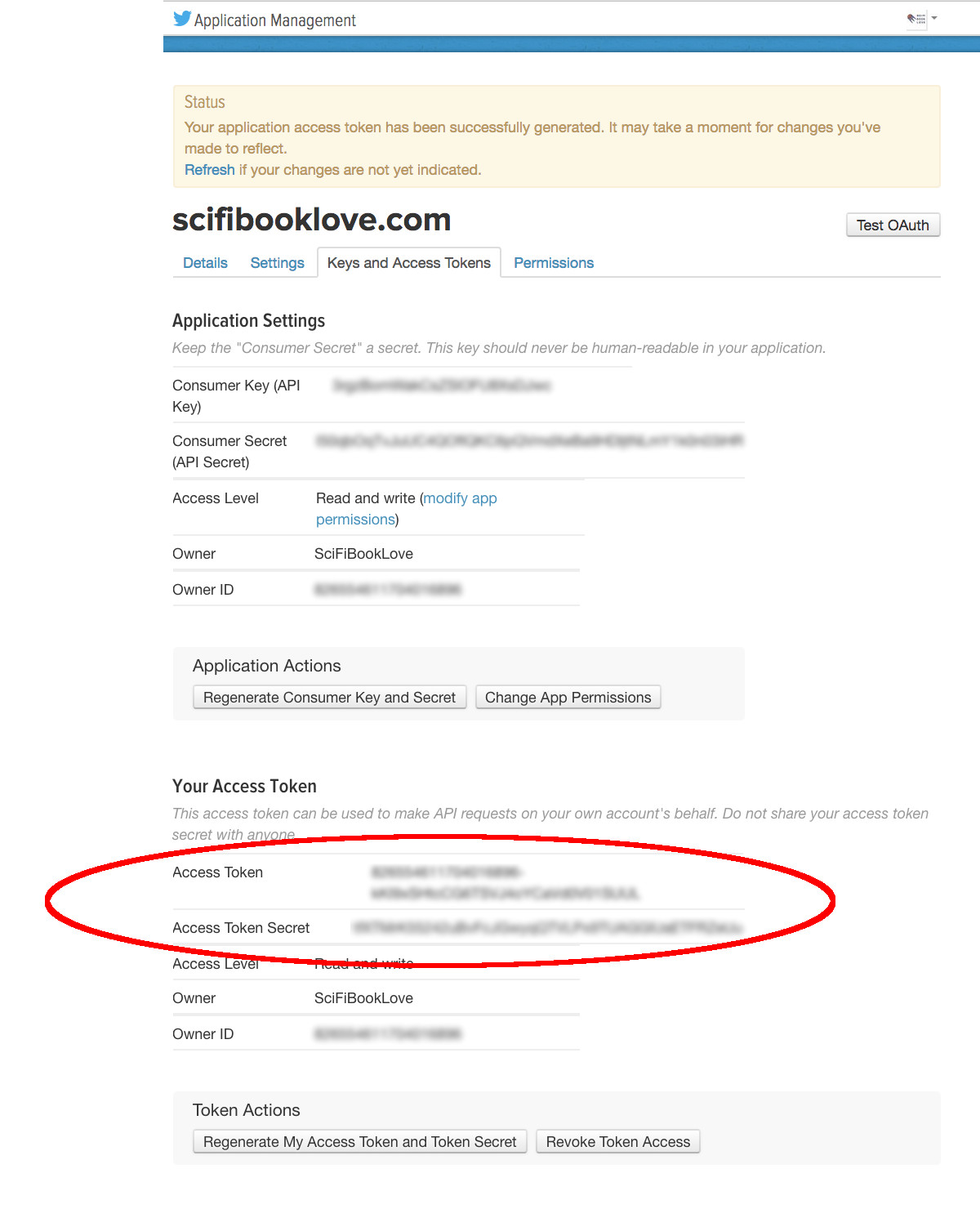
Step #2 - Set Up Python Dependencies
Now we need to create a directory for your python twitter posting code and add the dependencies you'll need.
Open up a command line terminal and follow along.
Note: I'm assuming you have python installed already. If not, head over to python.org's beginner's guide for instructions
First create a directory to store our twitter posting python code.
> mkdir simple_twitter_post
> cd simple_twitter_post
Now install a python virtual environment and activate it. If you haven't used python virtual environments before, read up here on why you should be.
> virtualenv venv --distribute
> source venv/bin/activate
Check if you're inside your virtual environment by echoing the bash environment variable $VIRTUAL_ENV
> echo $VIRTUAL_ENV
You should see something like this as a result...

We're going to be using the python package tweepy for doing the heavy lifting of interacting with Twitter's APIs. Install it now.
> pip install tweepy
You should see something like this as a result...
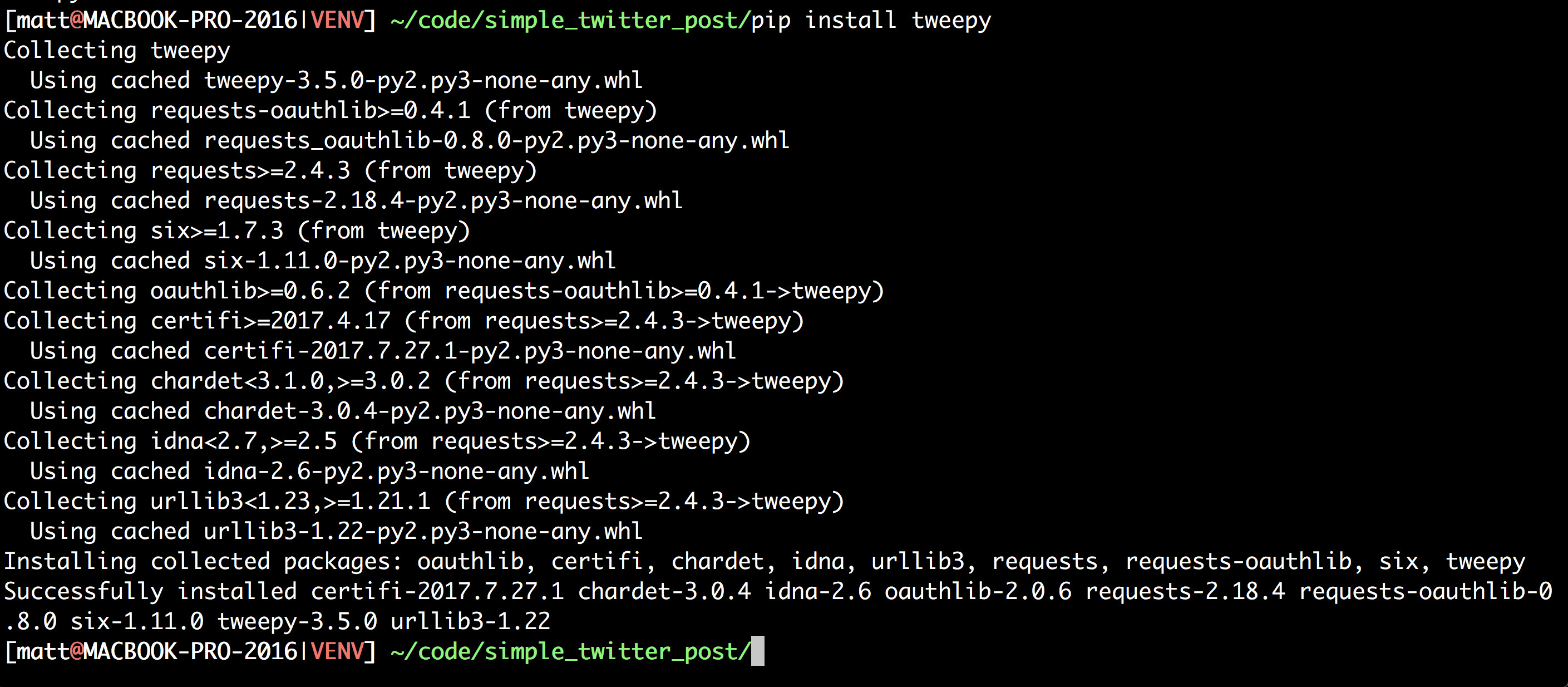
Check and make sure your package was installed by running pip freeze.
> pip freeze
You should see something like this as a result...
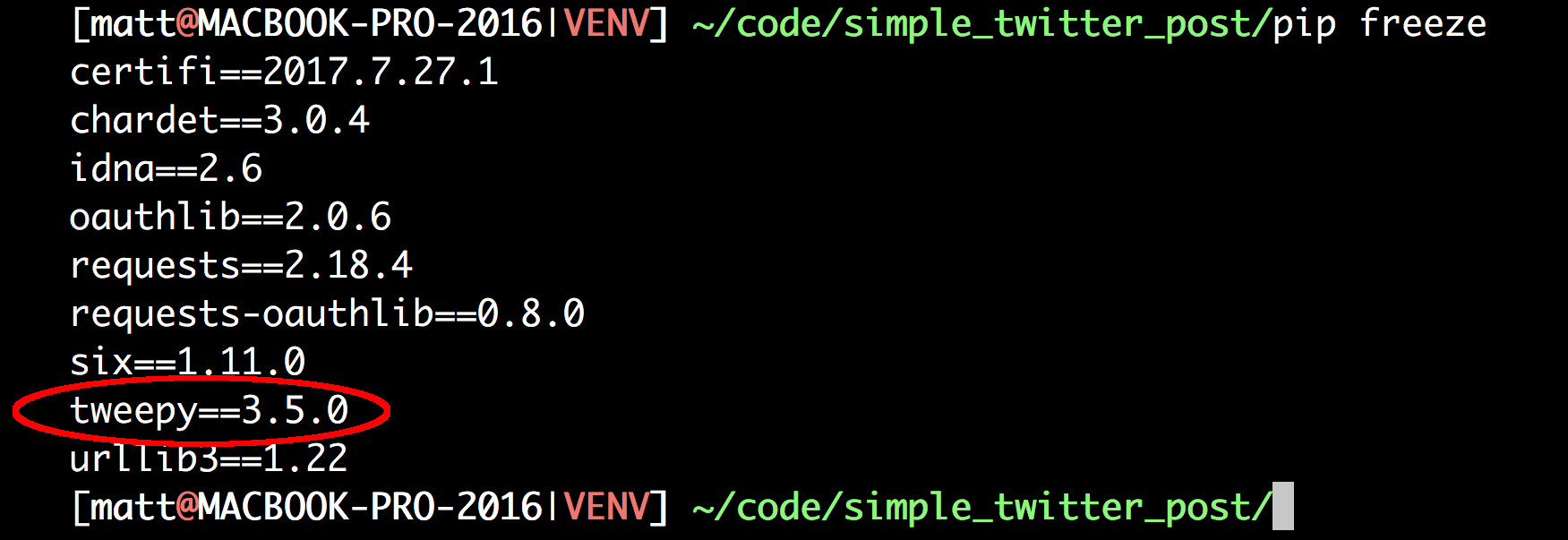
Step #3 - Write The Python Code
Ok, you have your python dependencies installed, we're ready to write our twitter posting code. Fire up your favorite code editor and follow along. VIM is mine (obv)
> vim post_tweet.py
Paste in the following code, make sure to substitute your keys and tokens you copied down from the earlier steps above.
import tweepy
def main():
twitter_auth_keys = {
"consumer_key" : "REPLACE_THIS_WITH_YOUR_CONSUMER_KEY",
"consumer_secret" : "REPLACE_THIS_WITH_YOUR_CONSUMER_SECRET",
"access_token" : "REPLACE_THIS_WITH_YOUR_ACCESS_TOKEN",
"access_token_secret" : "REPLACE_THIS_WITH_YOUR_ACCESS_TOKEN_SECRET"
}
auth = tweepy.OAuthHandler(
twitter_auth_keys['consumer_key'],
twitter_auth_keys['consumer_secret']
)
auth.set_access_token(
twitter_auth_keys['access_token'],
twitter_auth_keys['access_token_secret']
)
api = tweepy.API(auth)
tweet = "Another day, another #scifi #book and a cup of #coffee"
status = api.update_status(status=tweet)
if __name__ == "__main__":
main()
Step #4 - Post Your First Tweet
Now we're ready to run our code, fire away
> python post_tweet.py
If everything went right, you should see your brand new tweet on your twitter profile page.
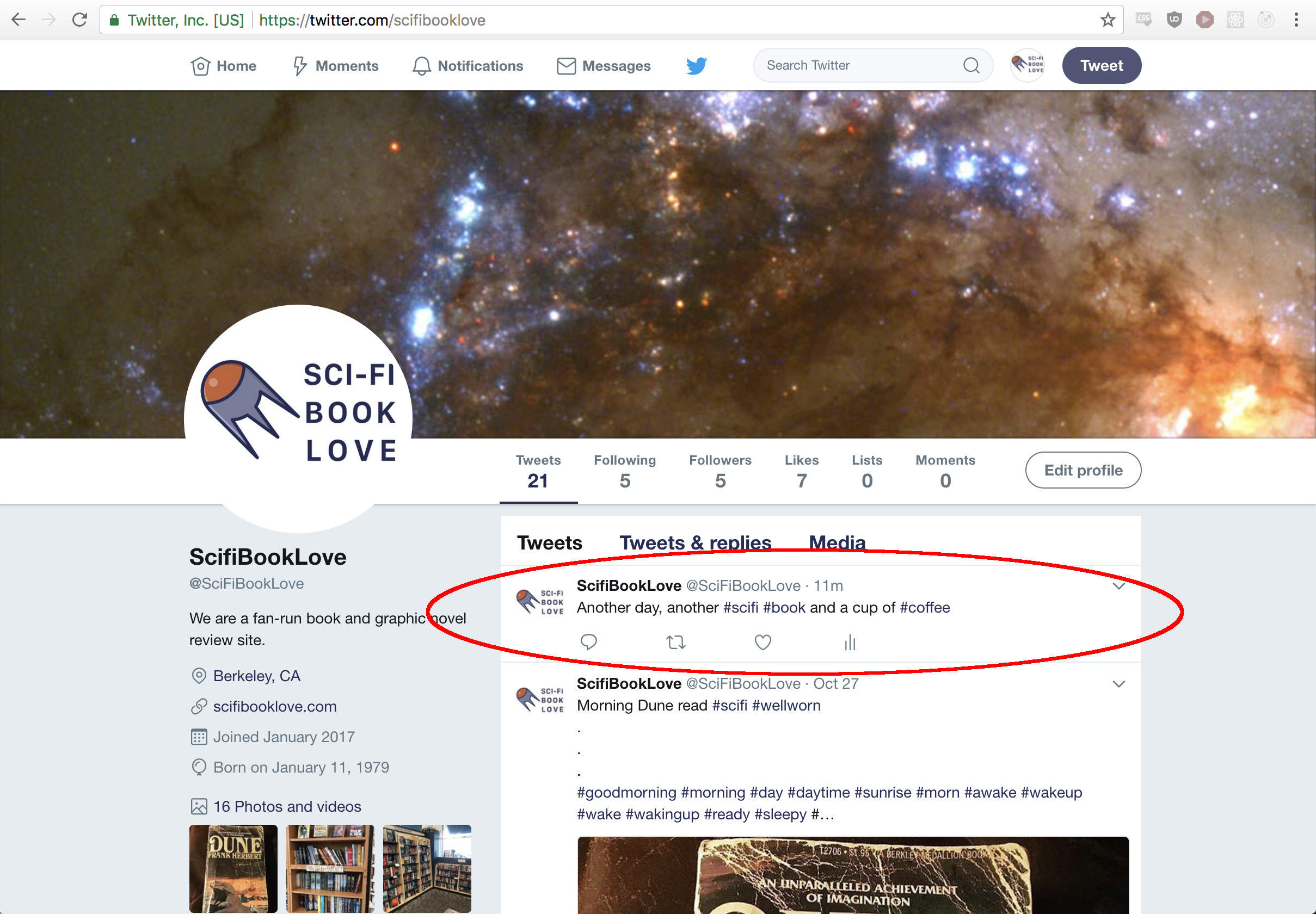
Thanks for reading, I hope you followed along and were able to get a version of this working for yourself. If you're having issues or want to chat, ping me on twitter at @mattccrampton
To: @mattccrampton
0
Other Posts
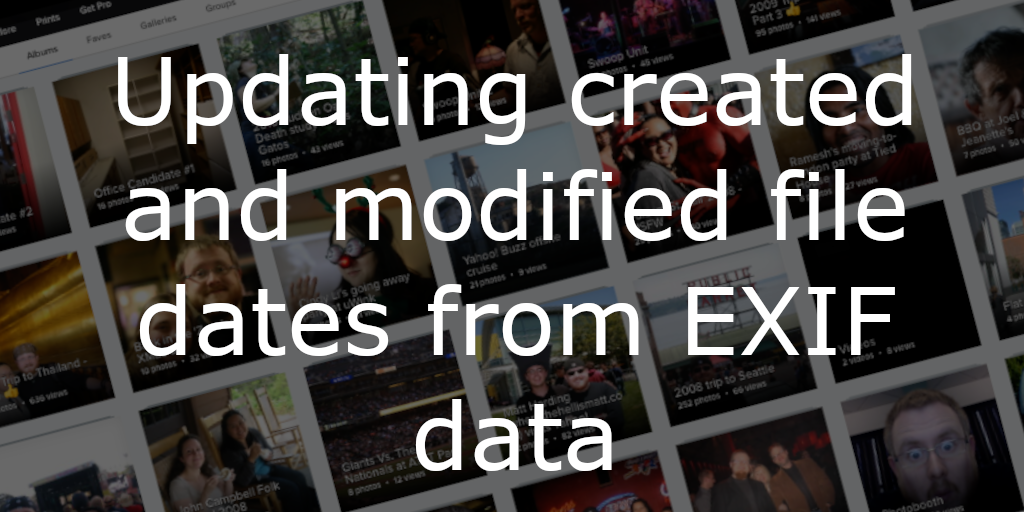
When exporting photos from a service like Flickr, perhaps after they've given notice that they're going to delete our photos if you don't subscribe to......
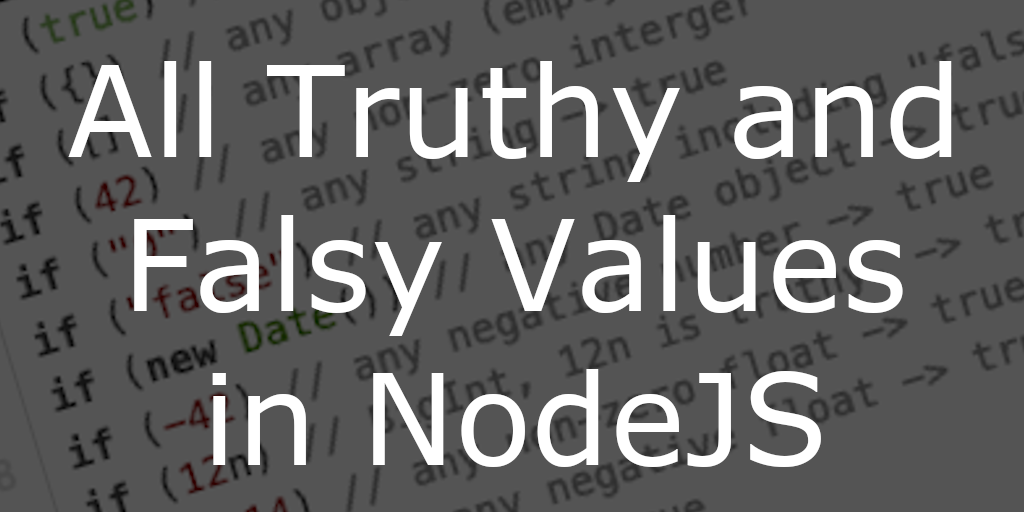
All Truthy and Falsy Javascript Values In Nodejs, every value has an associated boolean, true or false, value. For example, a null value has an......
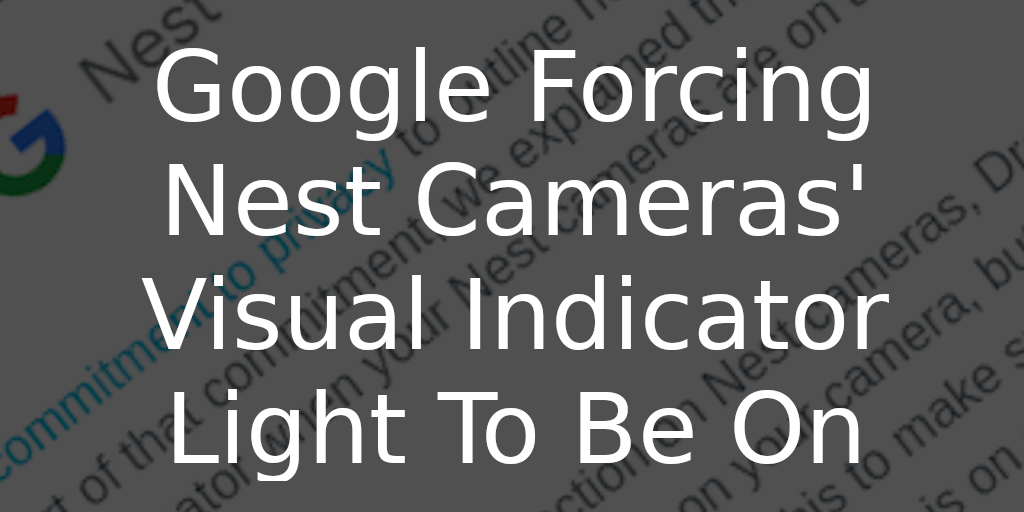
Google Forcing Nest Cameras Visual Indicator Light To Be On Received the following email from Google today... Full email text... Recently, we shared our commitment......

Posting to Twitter with Python - Part Two: Posting Photos NOTE: This is part two of my posting to Twitter with Python tutorial. If you......
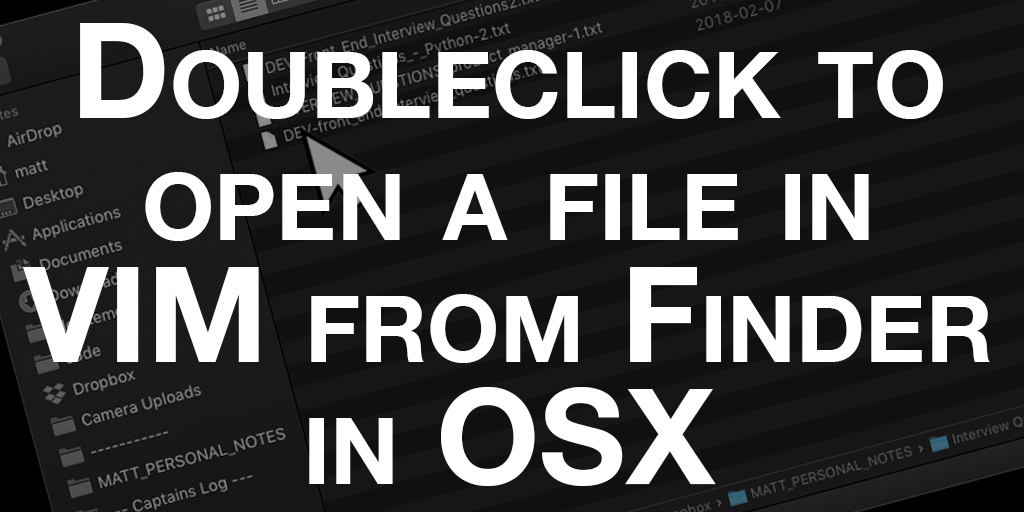
Doubleclick to open a file in VIM from OSX I use VIM for just about everything from note taking to coding to keeping track of......
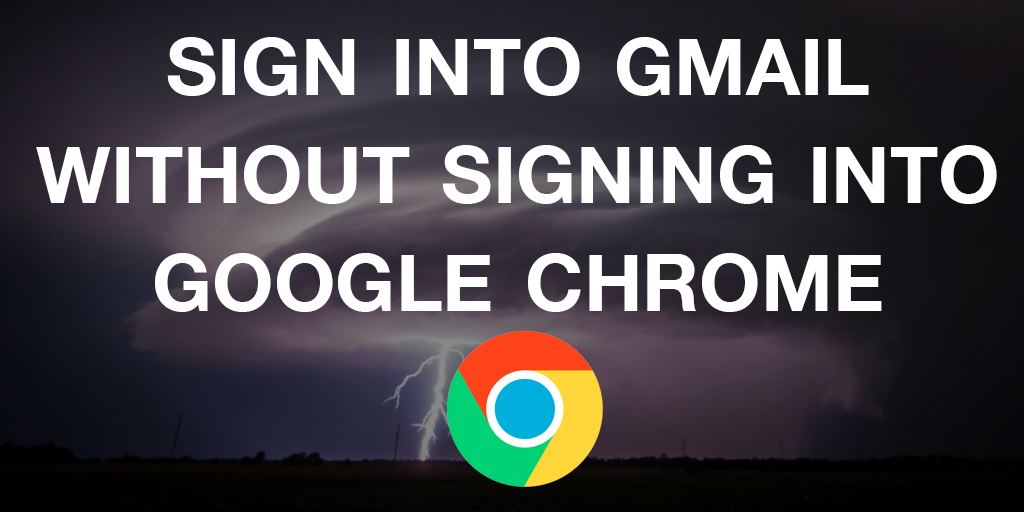
Sign Into Gmail Without Signing Into Google Chrome Unfortunately, Google has made changes to Chrome since this blog post was posted which removed the options......
Other Software Development Blog Posts
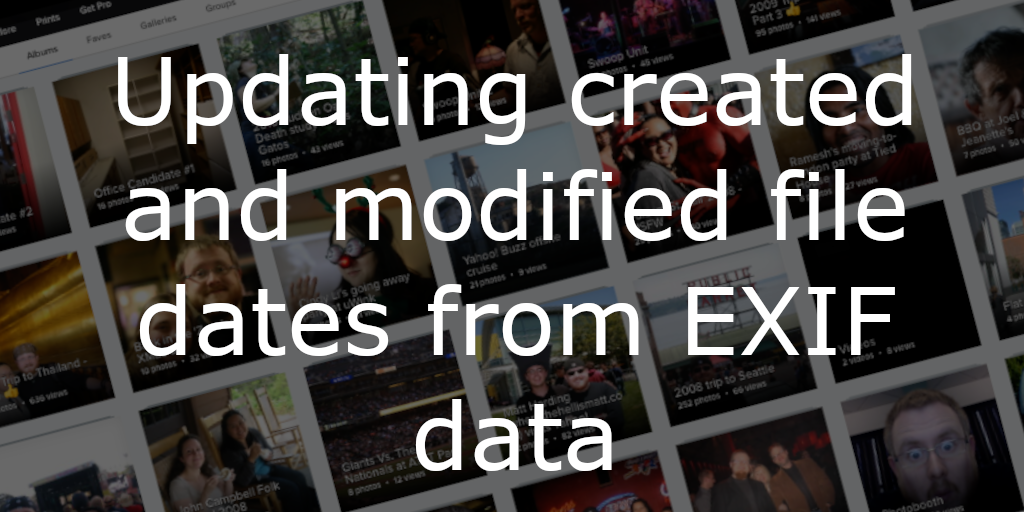
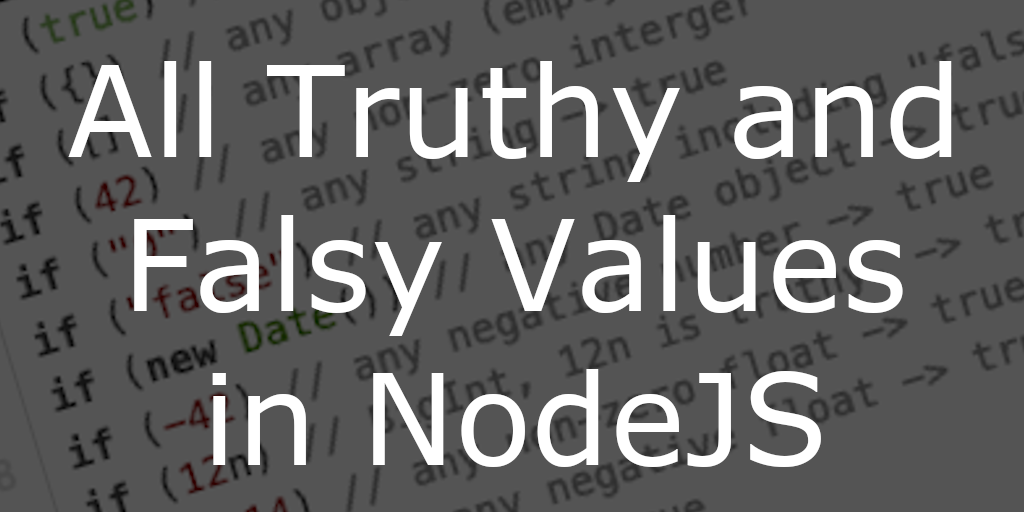
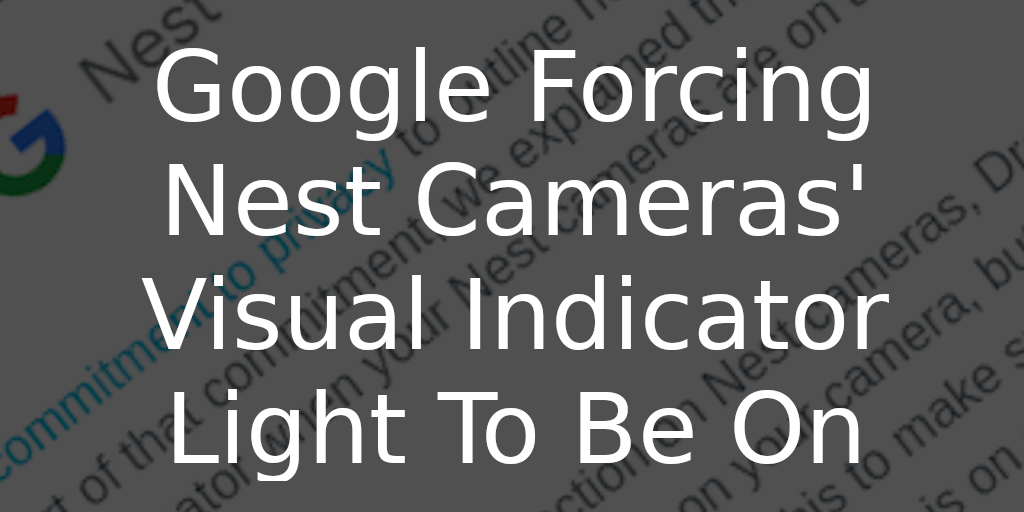

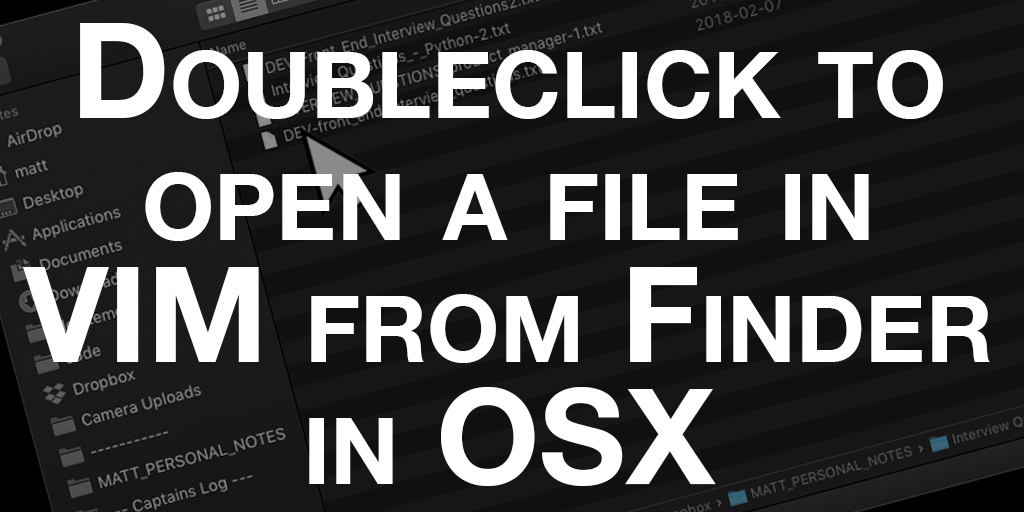
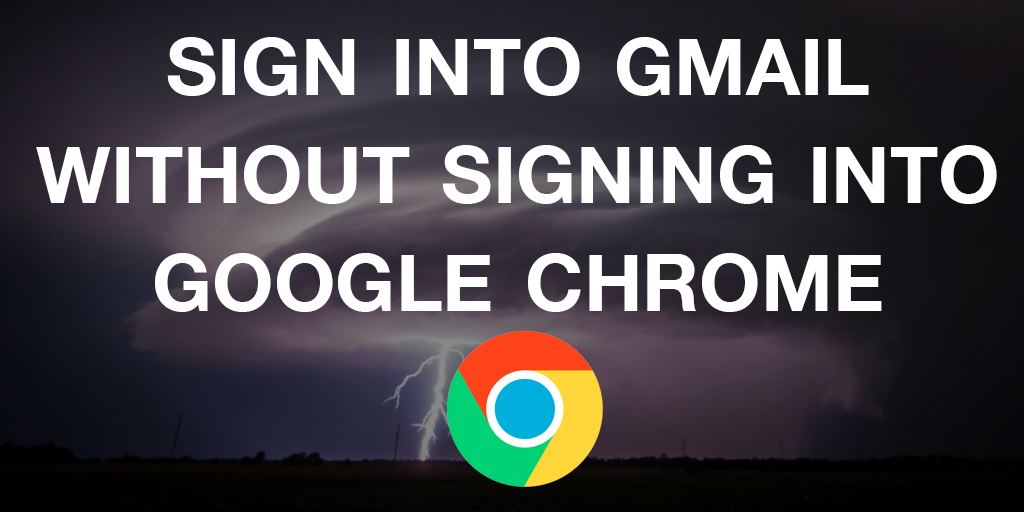
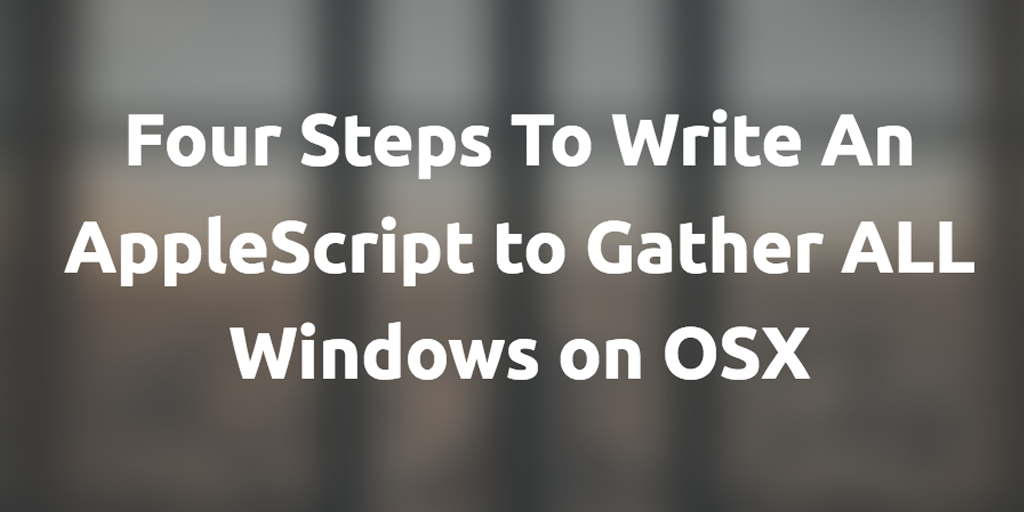
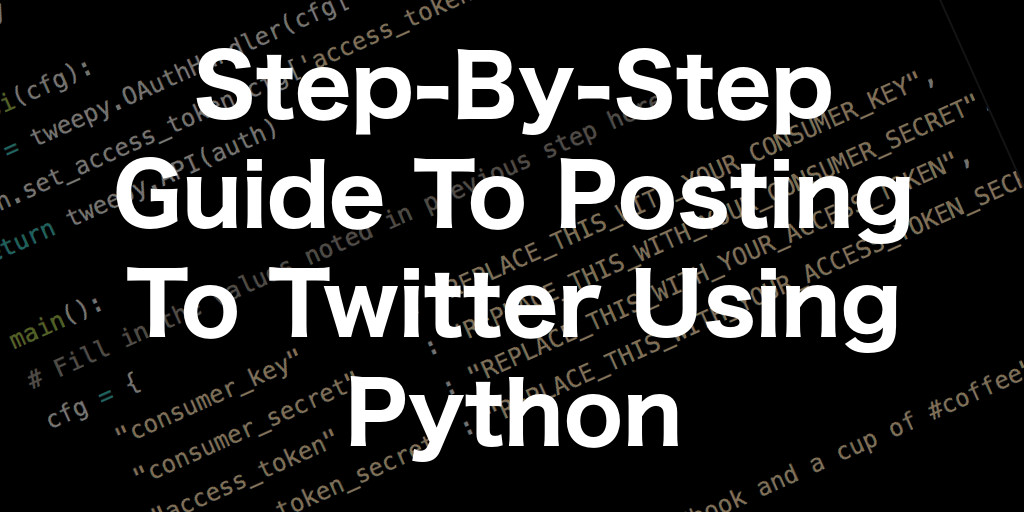
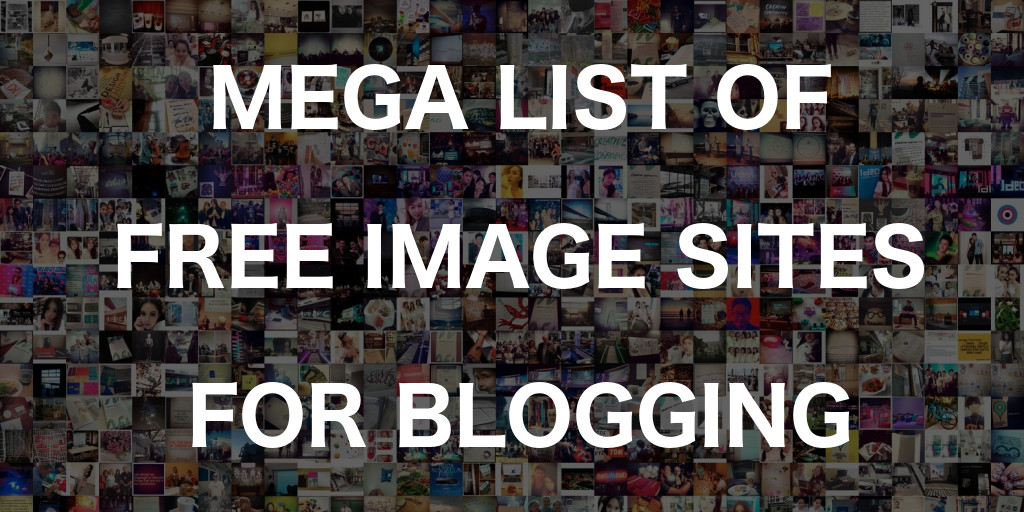
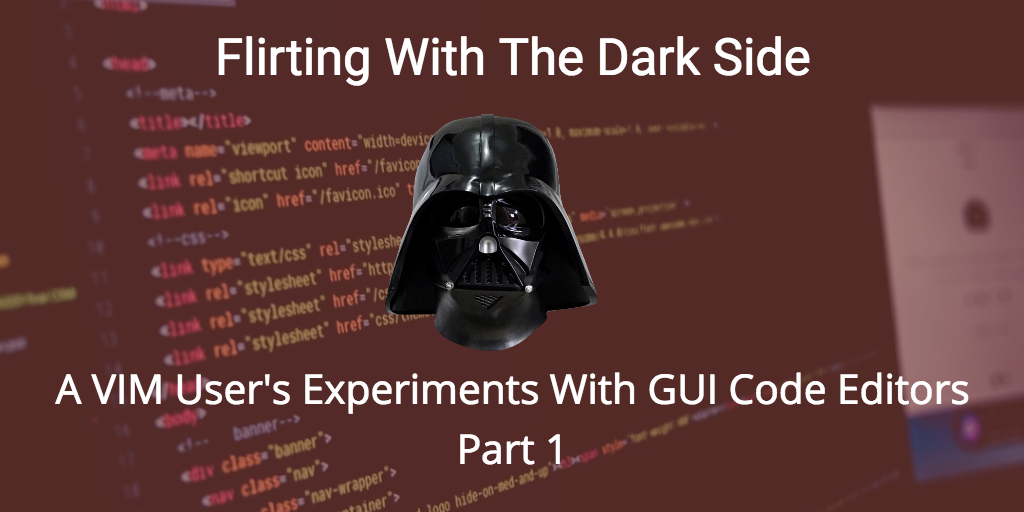
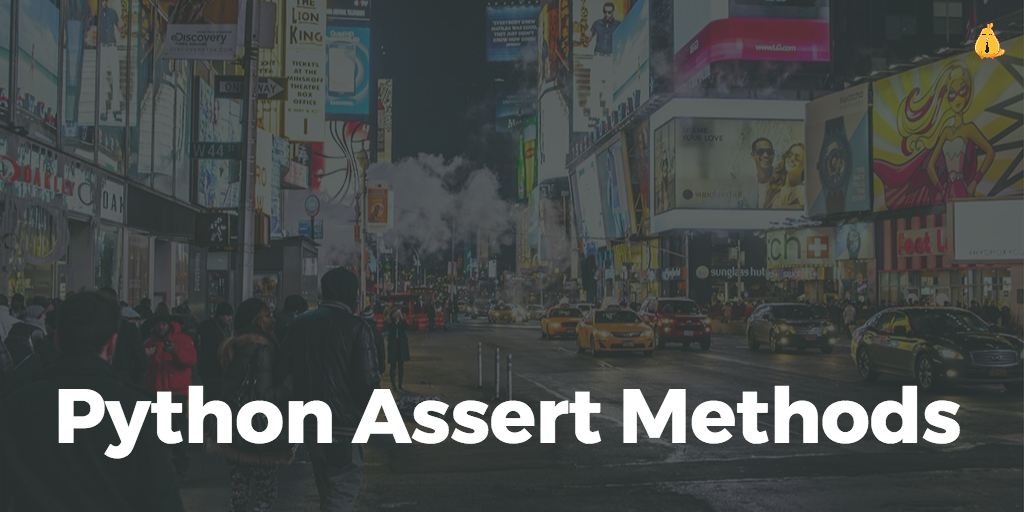
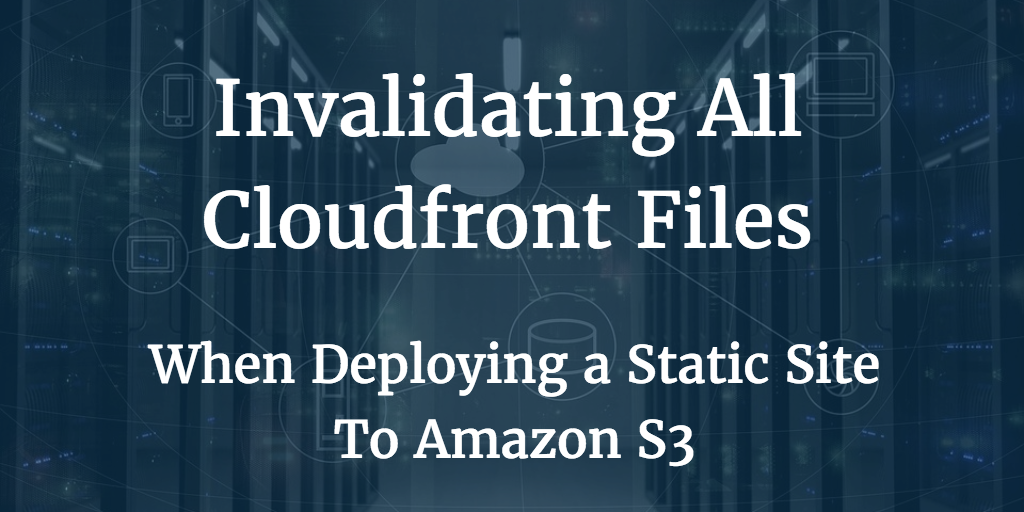
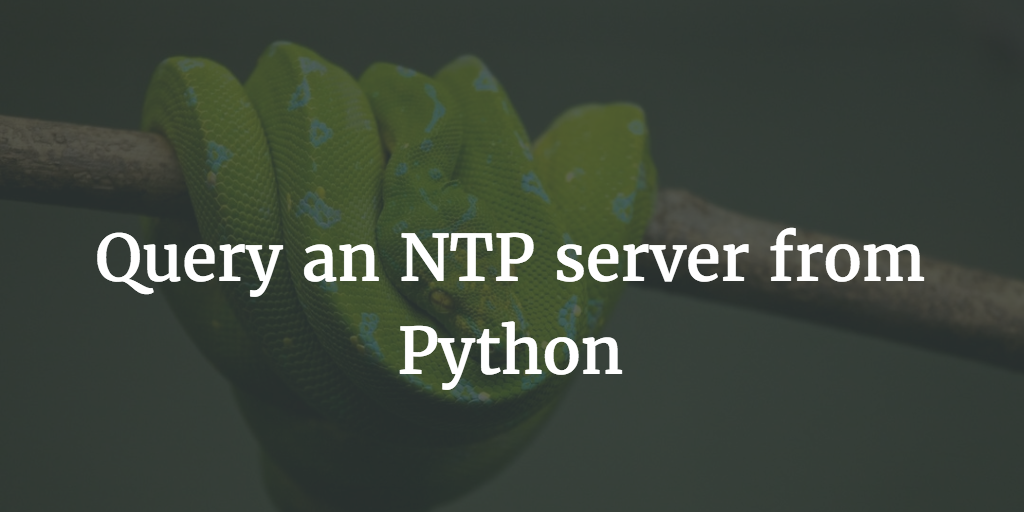
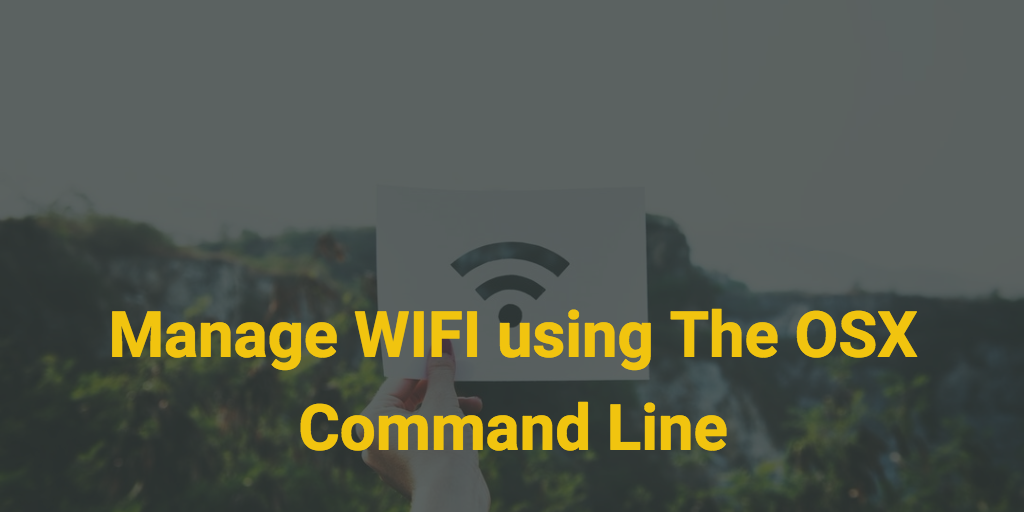
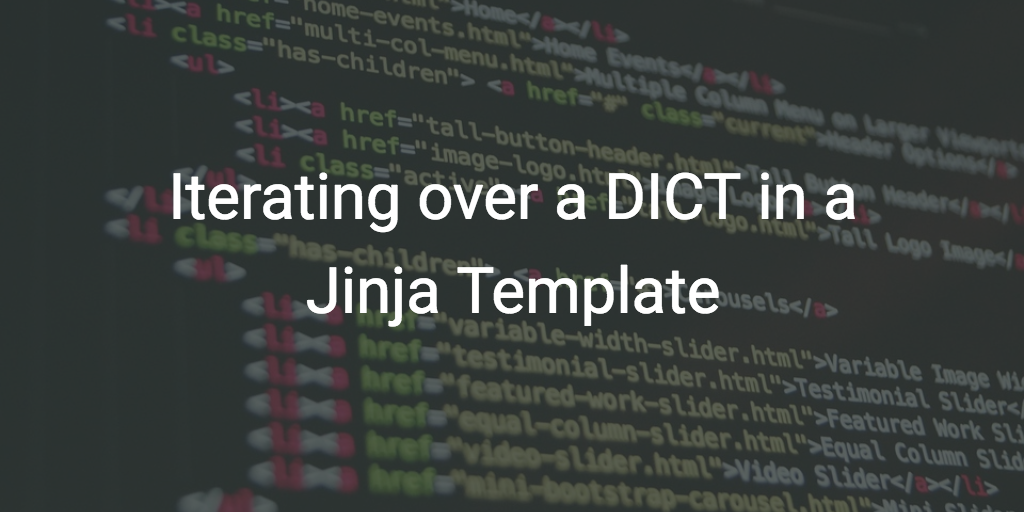
Other Blog Posts
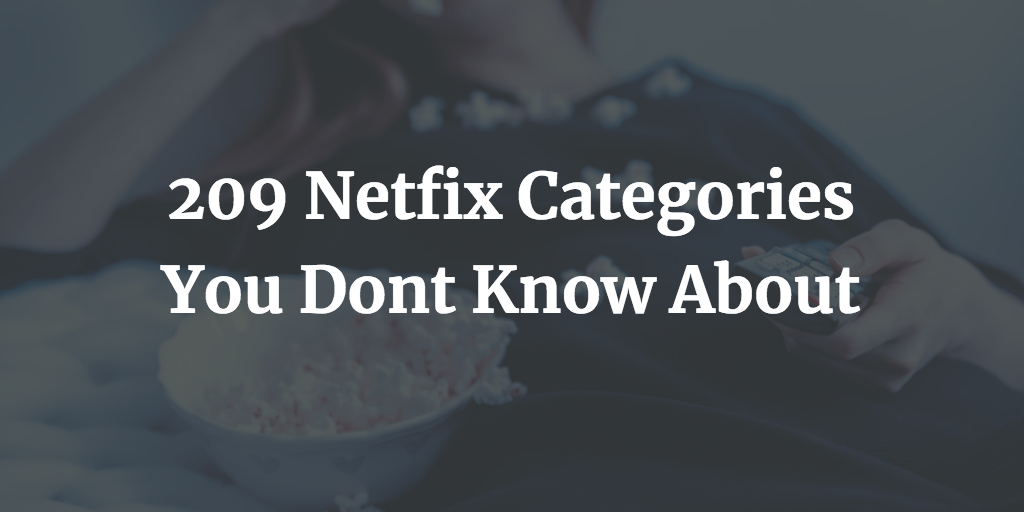
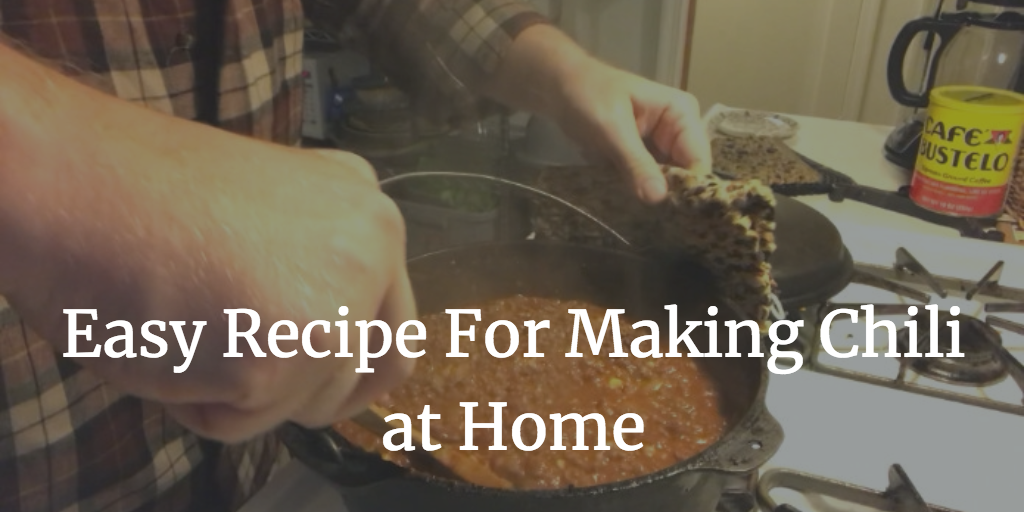
Media Appearances
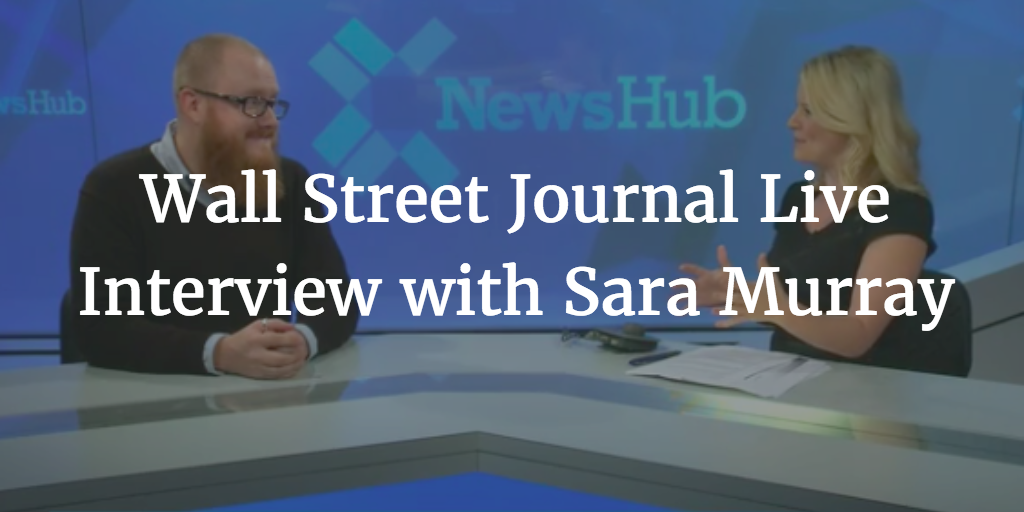
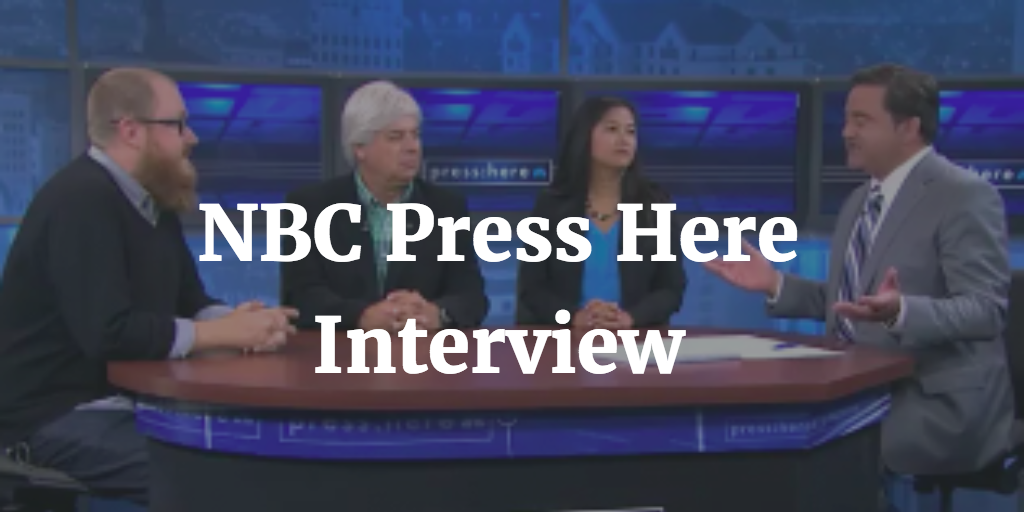
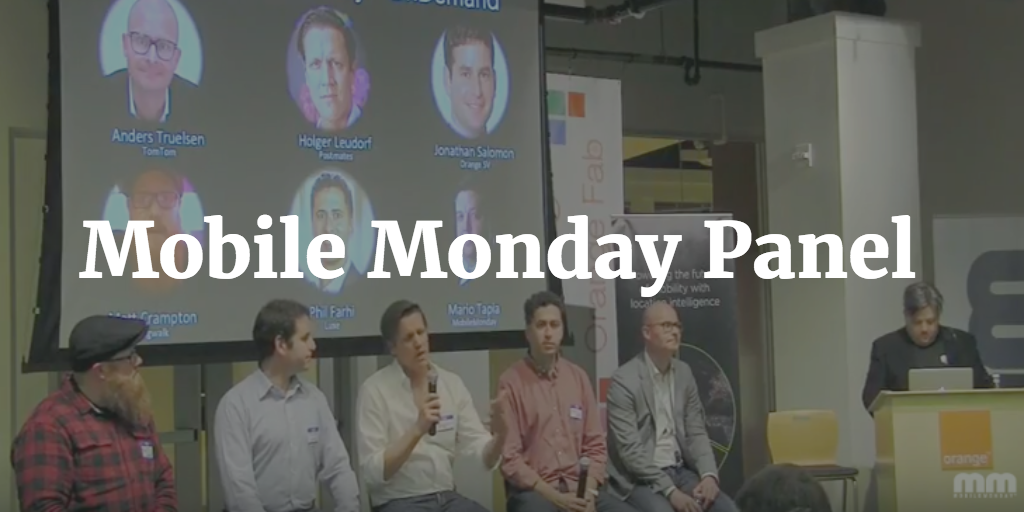
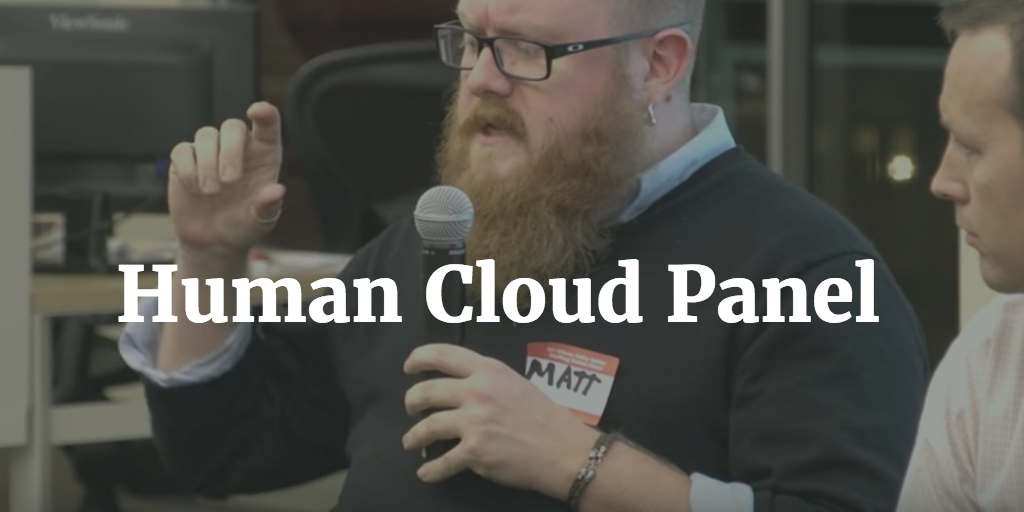
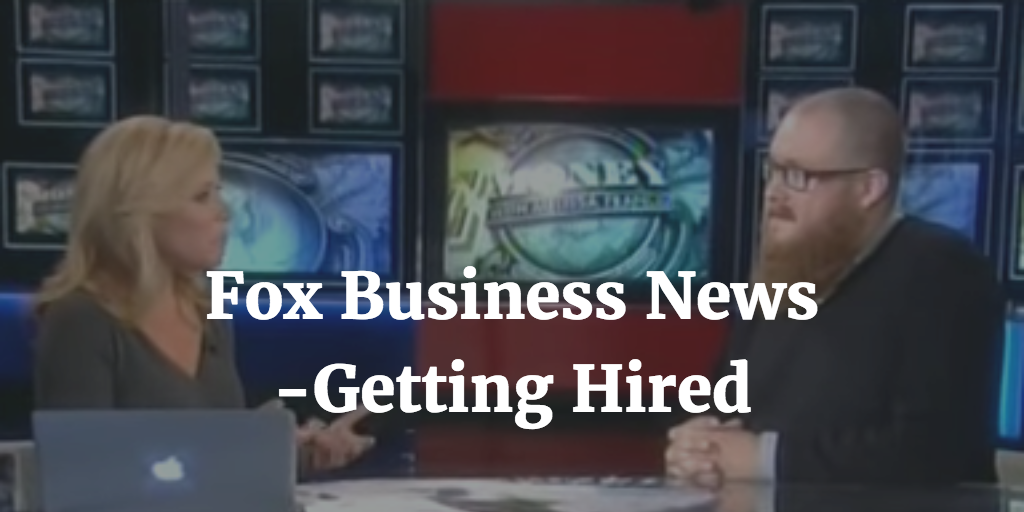
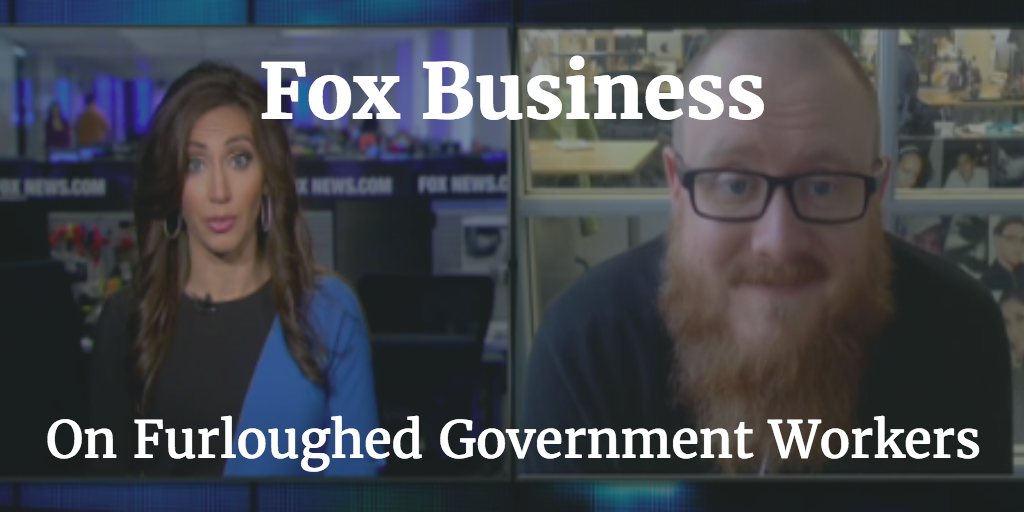
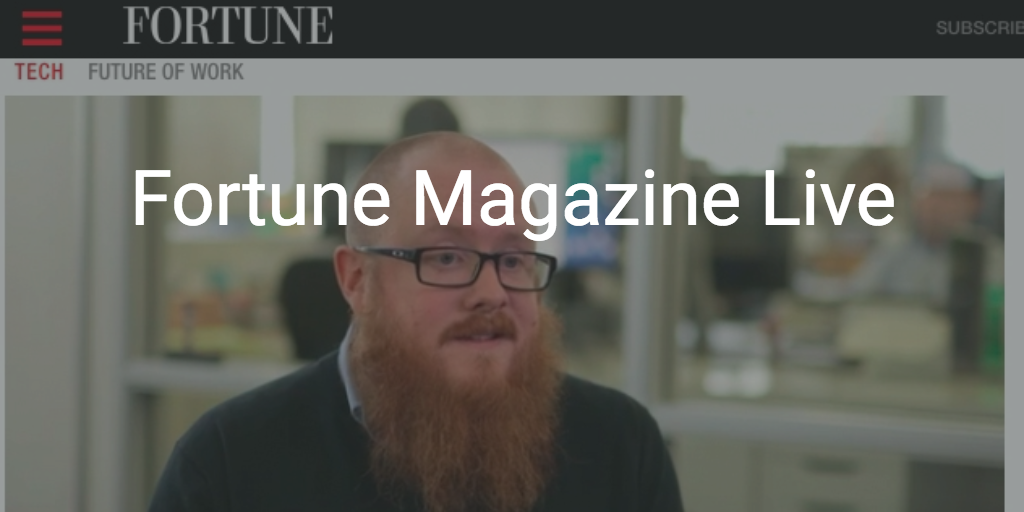
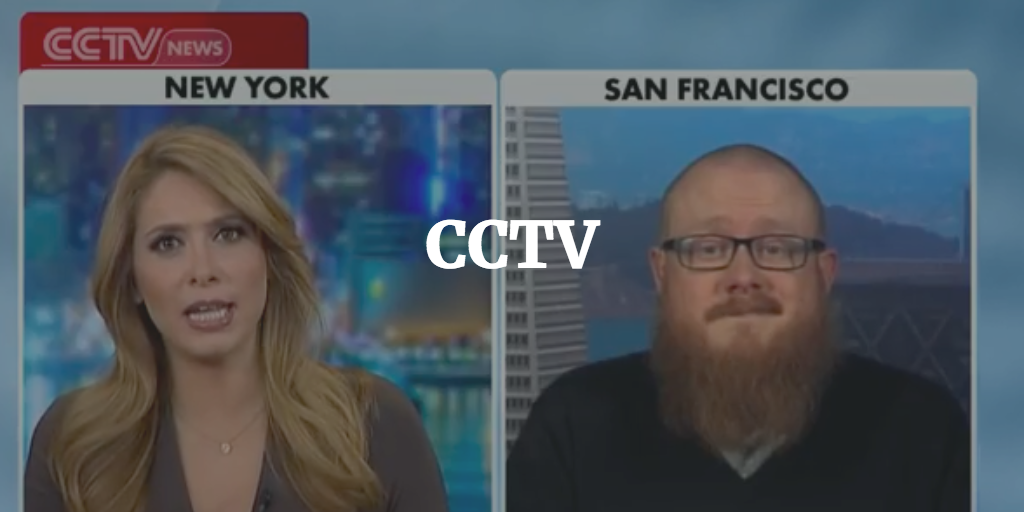
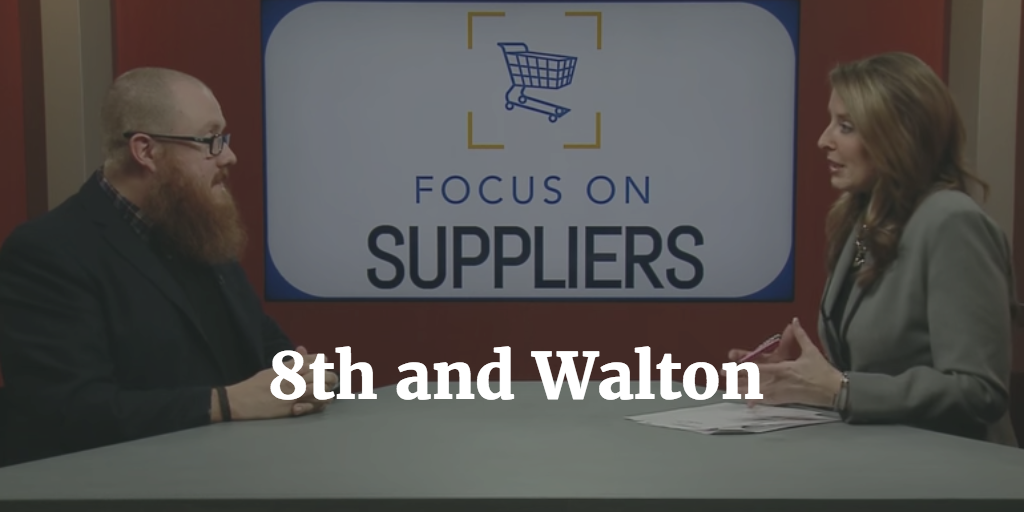
Print Interviews

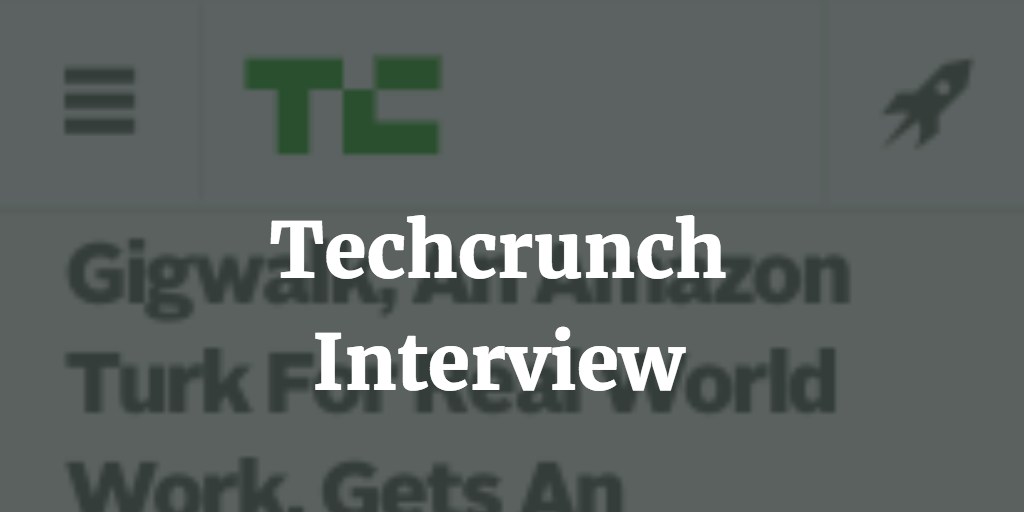
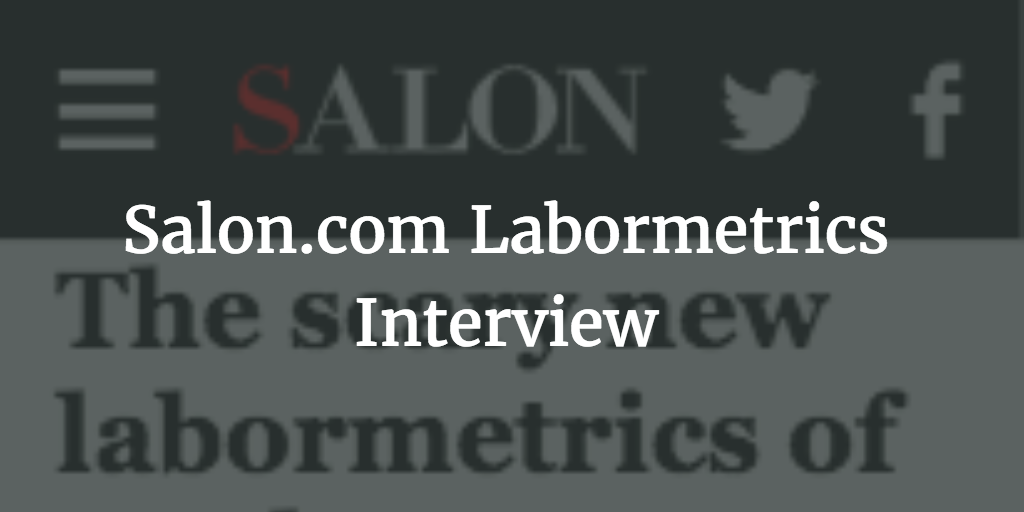
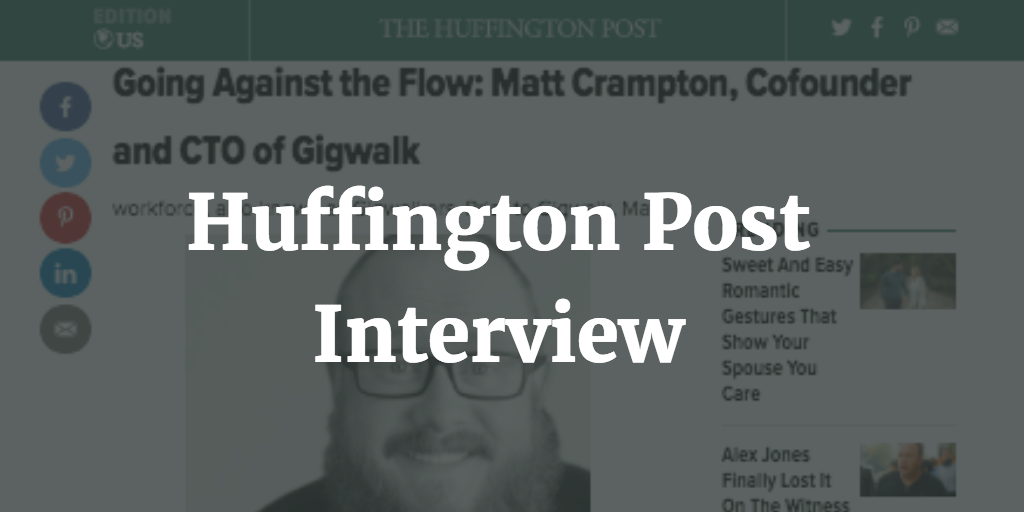
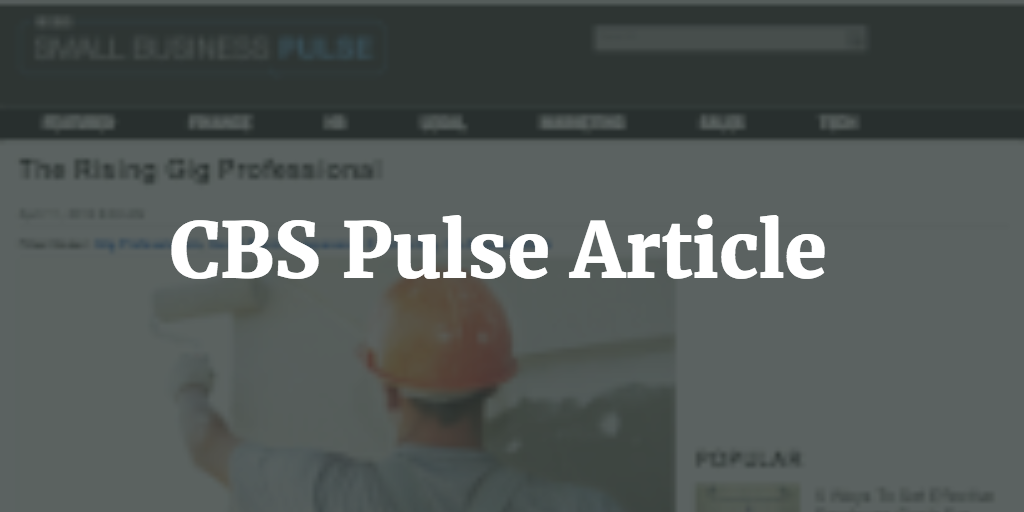
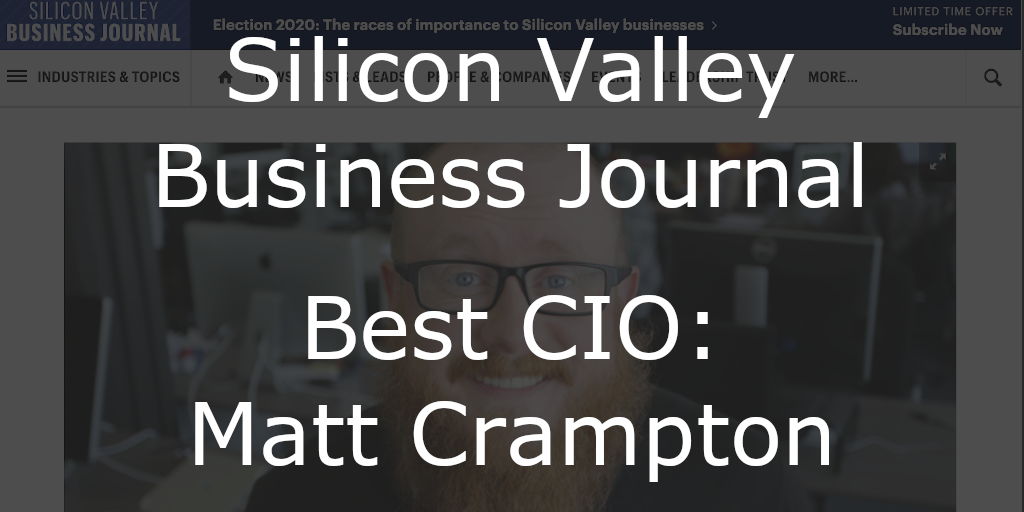