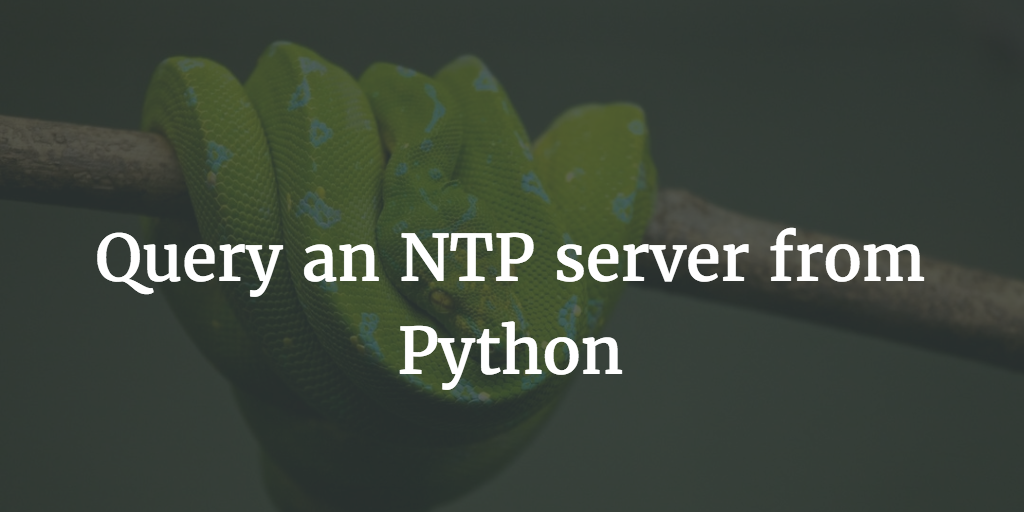
Query an NTP server from Python
I came across a need to query an
NTP
server to get the current time from a python
script. There are some great python modules you can install which will
take care of the heavy lifting (one great one is ntplib),
but I wanted to be able to do these NTP queries without requiring the host
to install 3rd party python packages.
As it turns out, it wasn't too difficult after checking out a few stack overflow threads, I put together this little script...
Python Pull NPT Time Script
#!/usr/bin/env python
from socket import AF_INET, SOCK_DGRAM
import sys
import socket
import struct, time
def getNTPTime(host = "pool.ntp.org"):
port = 123
buf = 1024
address = (host,port)
msg = '\x1b' + 47 * '\0'
# reference time (in seconds since 1900-01-01 00:00:00)
TIME1970 = 2208988800 # 1970-01-01 00:00:00
# connect to server
client = socket.socket( AF_INET, SOCK_DGRAM)
client.sendto(msg.encode('utf-8'), address)
msg, address = client.recvfrom( buf )
t = struct.unpack( "!12I", msg )[10]
t -= TIME1970
return time.ctime(t).replace(" "," ")
if __name__ == "__main__":
print(getNTPTime())
Create a file, paste that code in, chmod +x it and fire it off.
To: @mattccrampton
0
Other Posts
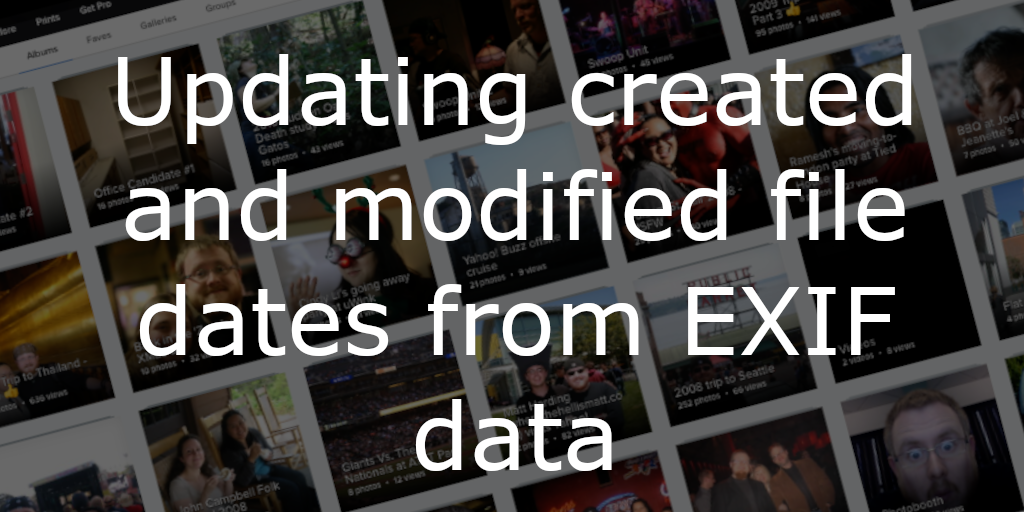
When exporting photos from a service like Flickr, perhaps after they've given notice that they're going to delete our photos if you don't subscribe to......
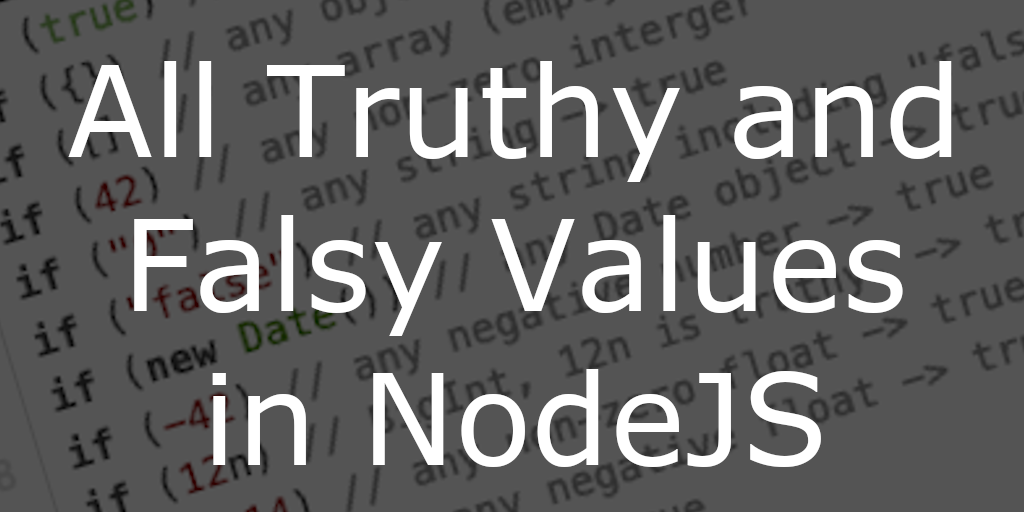
All Truthy and Falsy Javascript Values In Nodejs, every value has an associated boolean, true or false, value. For example, a null value has an......
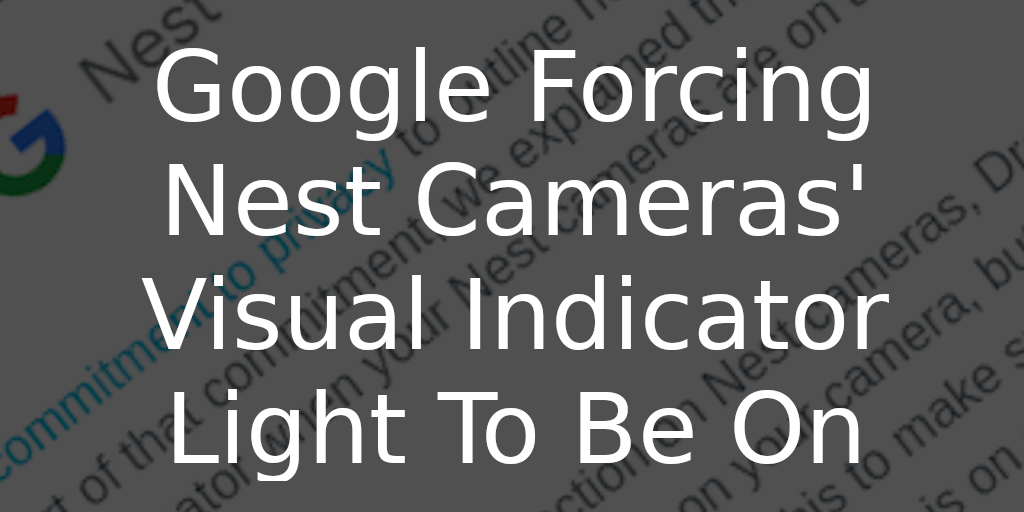
Google Forcing Nest Cameras Visual Indicator Light To Be On Received the following email from Google today... Full email text... Recently, we shared our commitment......

Posting to Twitter with Python - Part Two: Posting Photos NOTE: This is part two of my posting to Twitter with Python tutorial. If you......
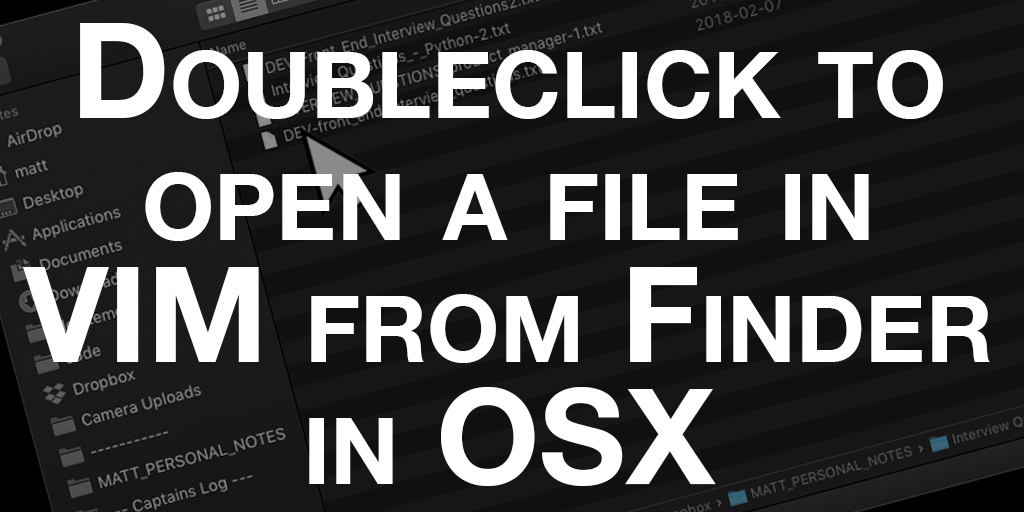
Doubleclick to open a file in VIM from OSX I use VIM for just about everything from note taking to coding to keeping track of......
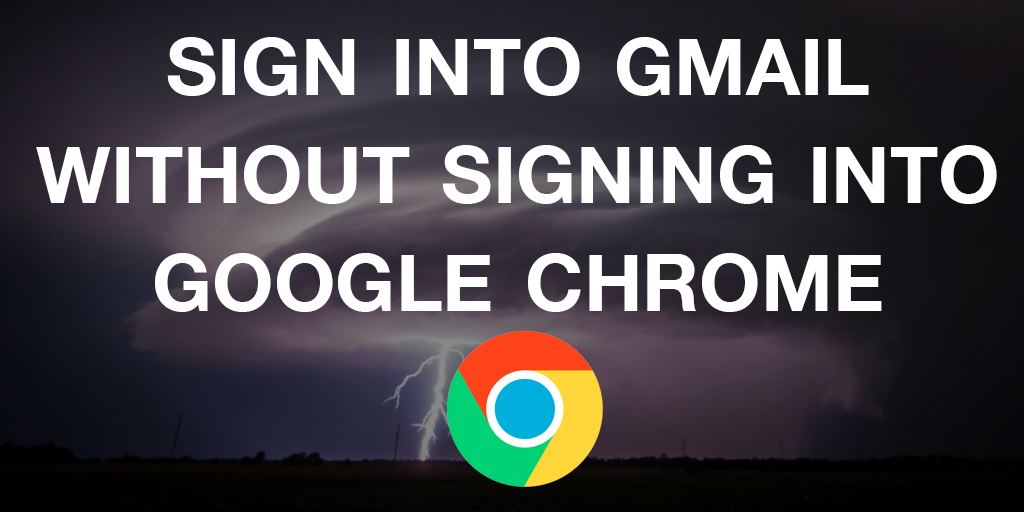
Sign Into Gmail Without Signing Into Google Chrome Unfortunately, Google has made changes to Chrome since this blog post was posted which removed the options......
Other Software Development Blog Posts
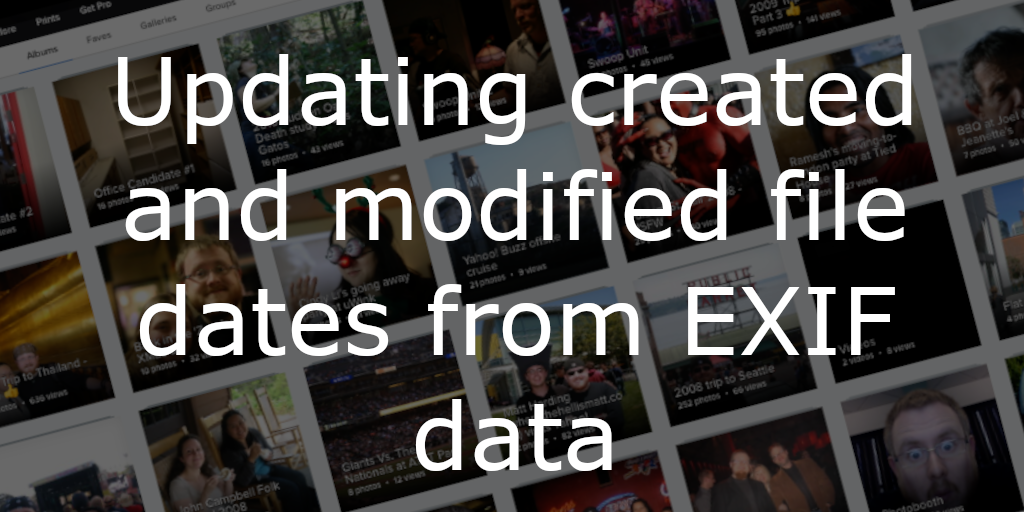
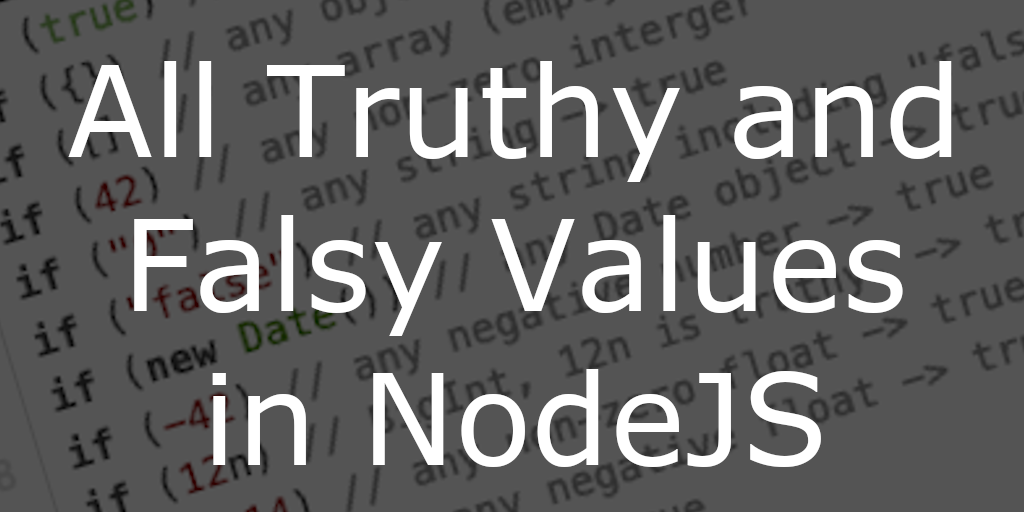
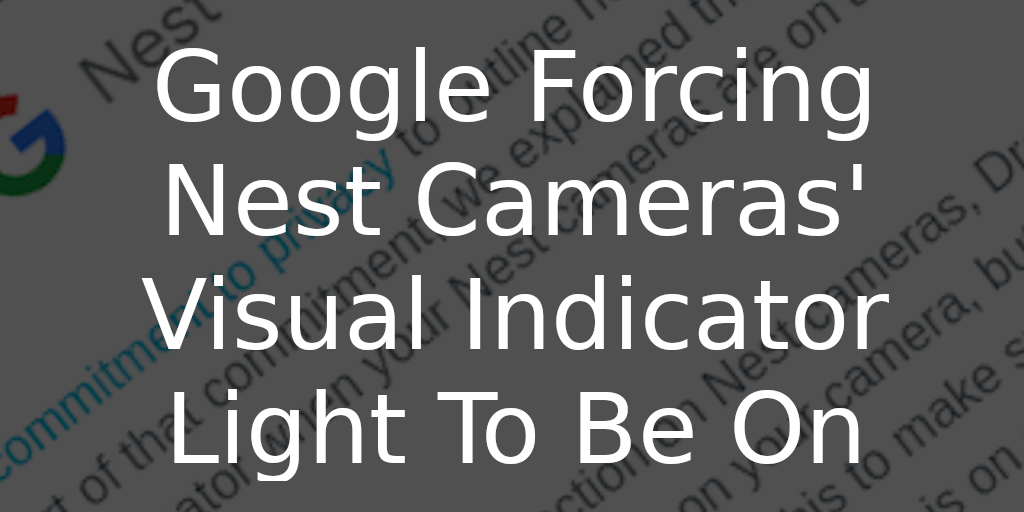

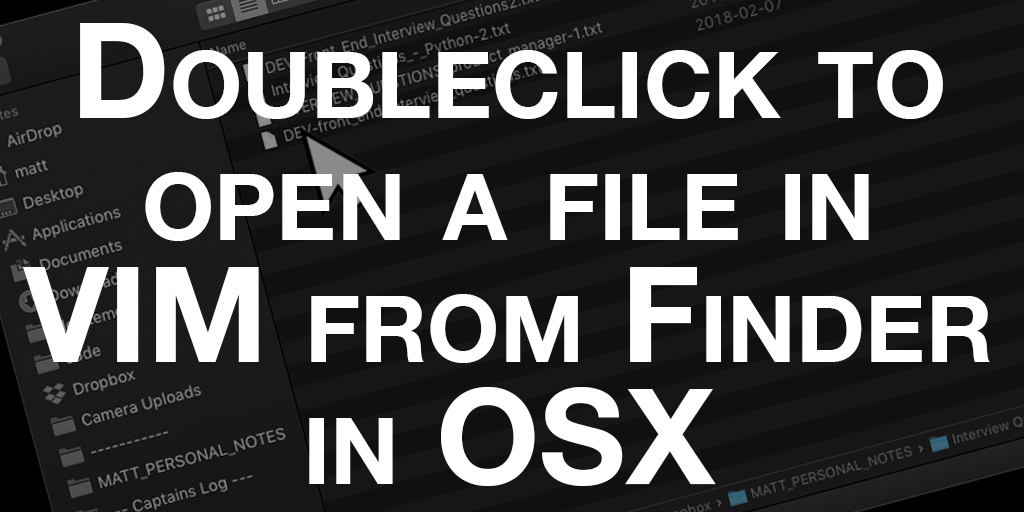
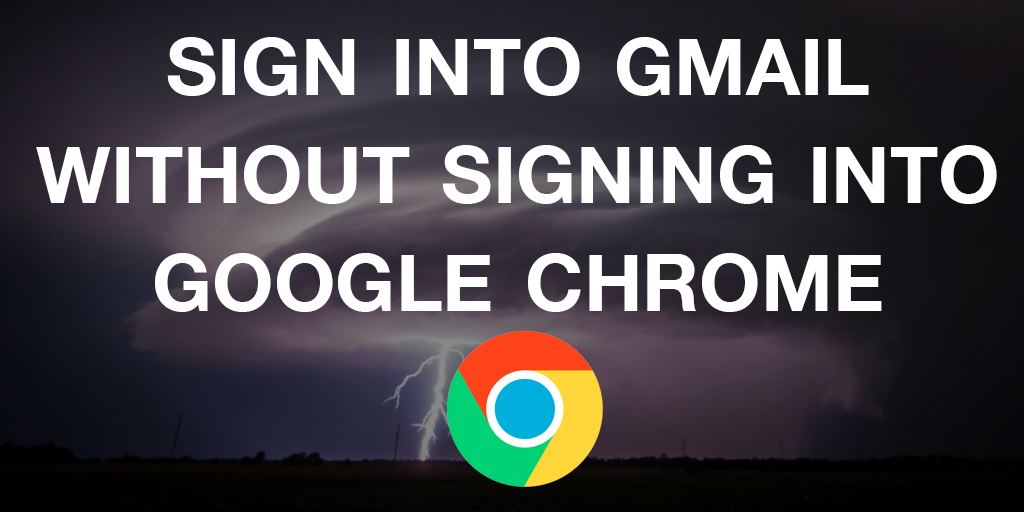
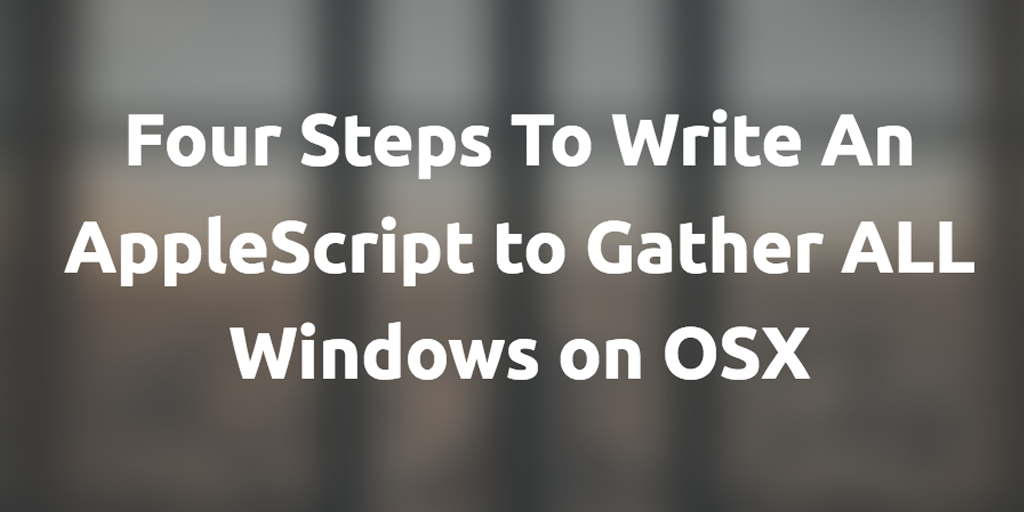
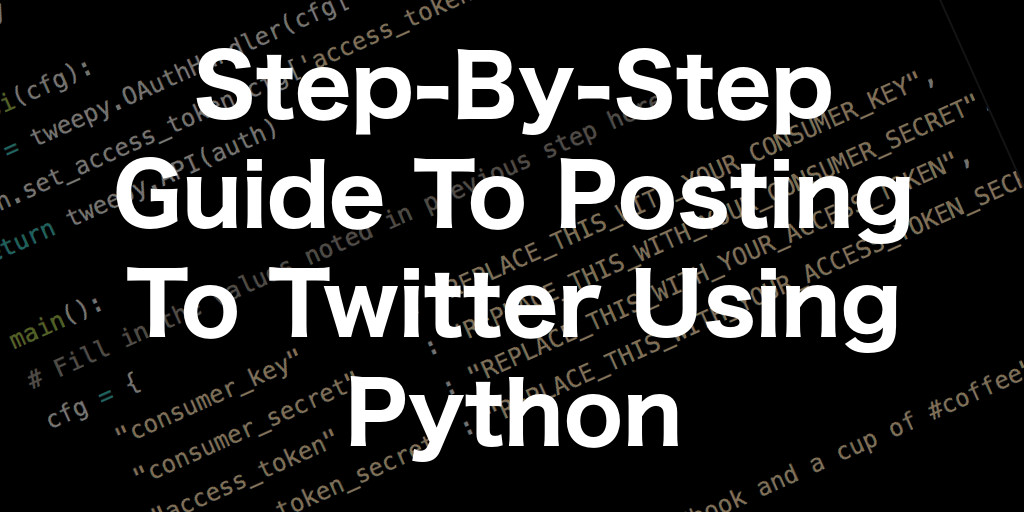
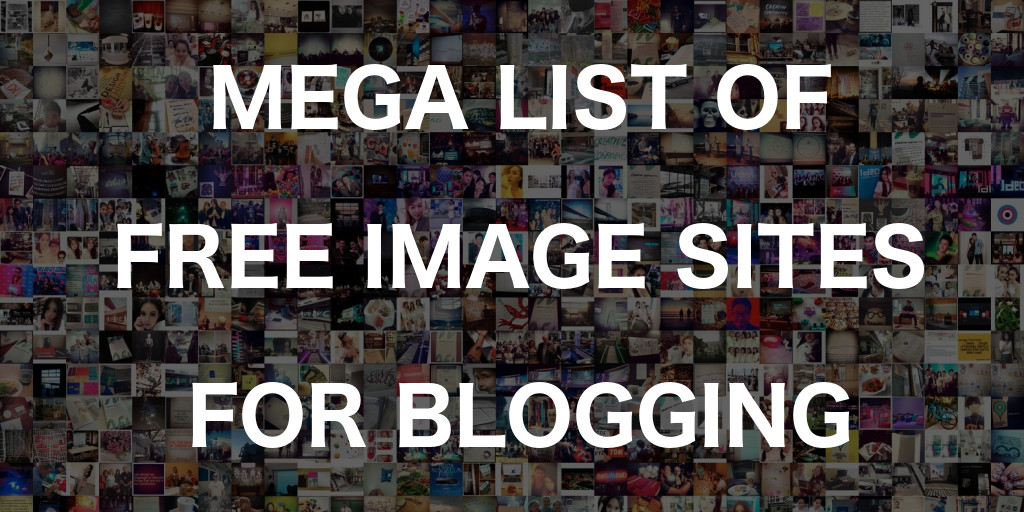
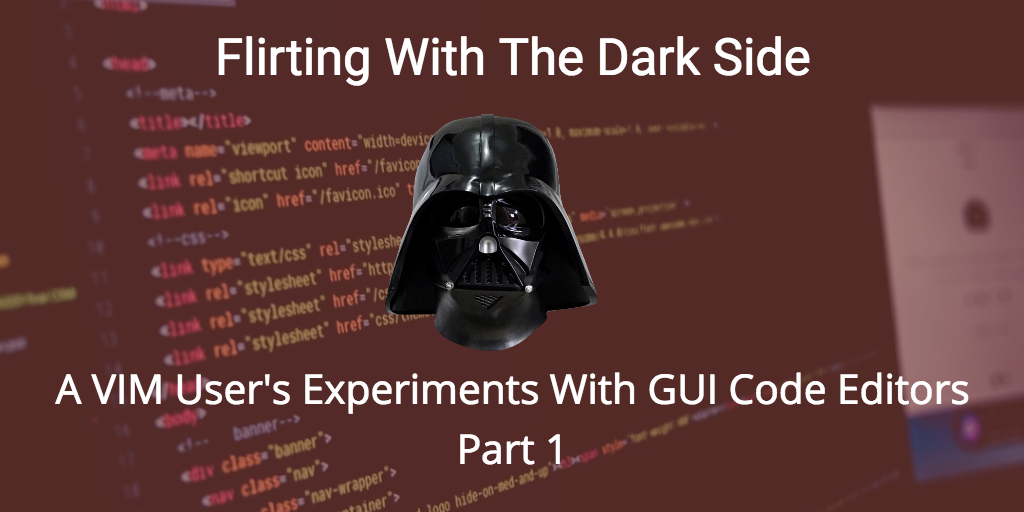
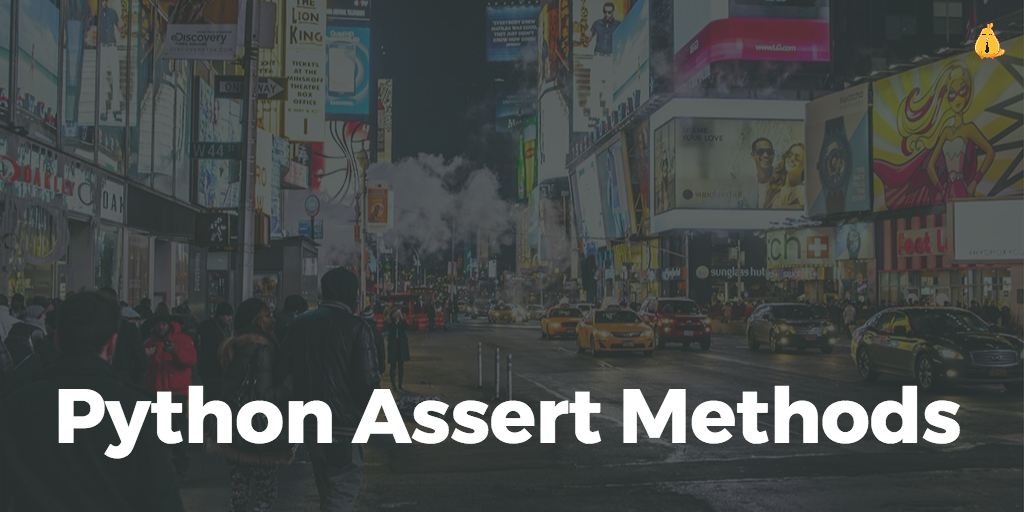
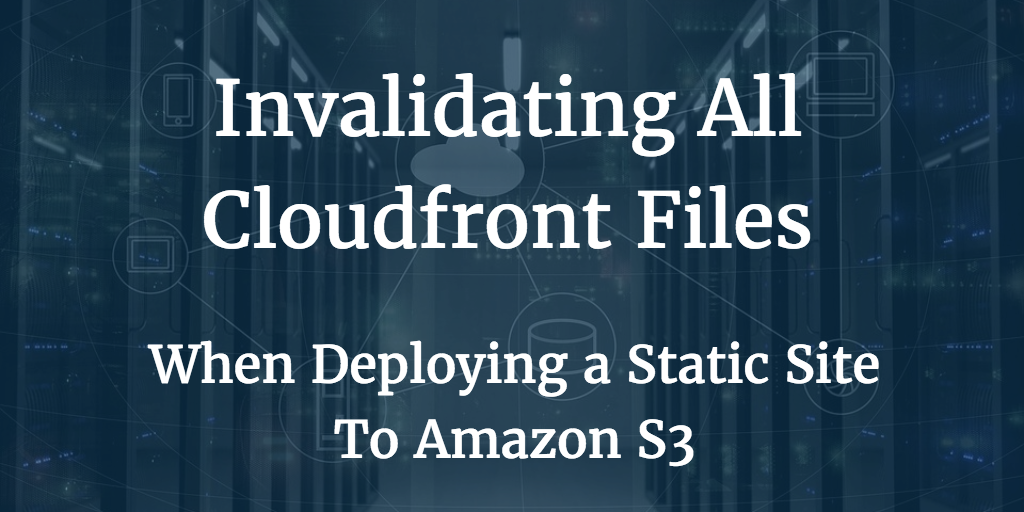
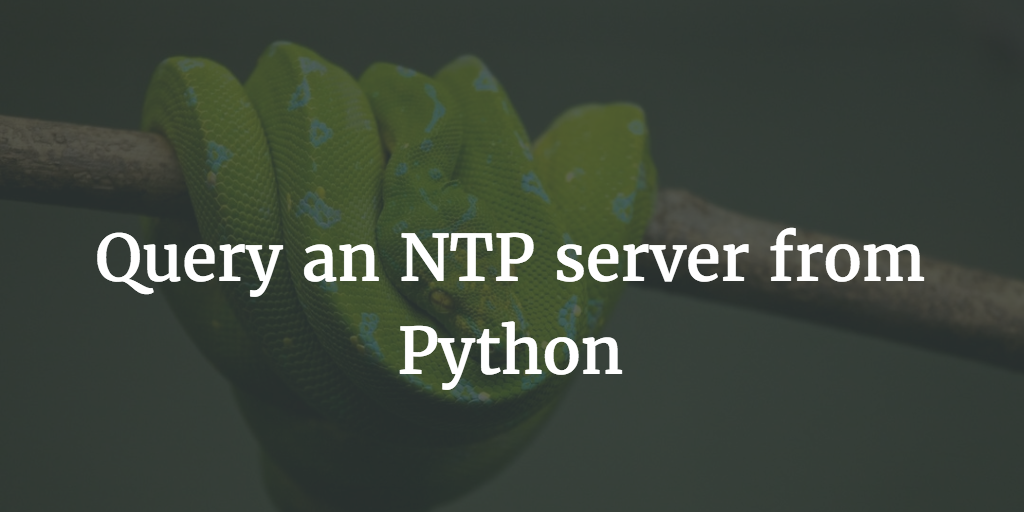
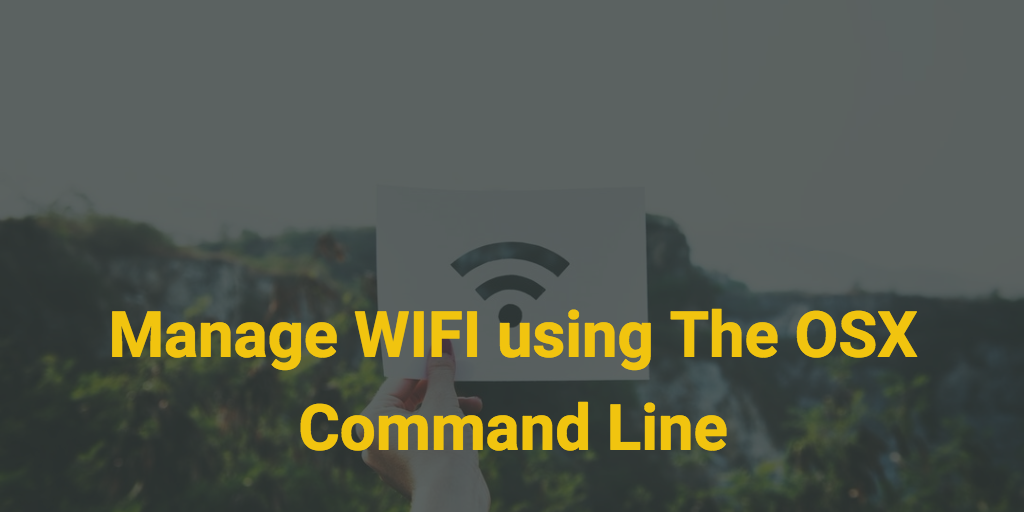
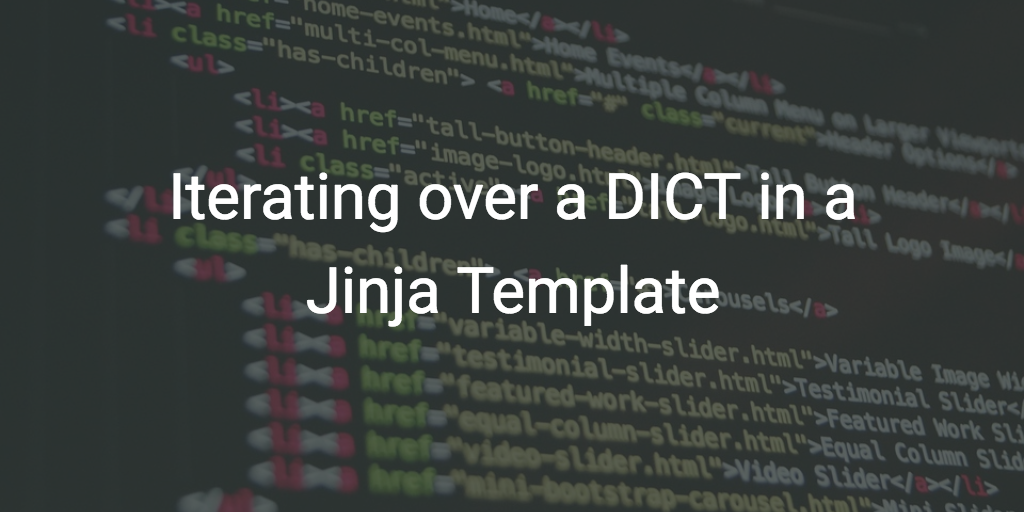
Other Blog Posts
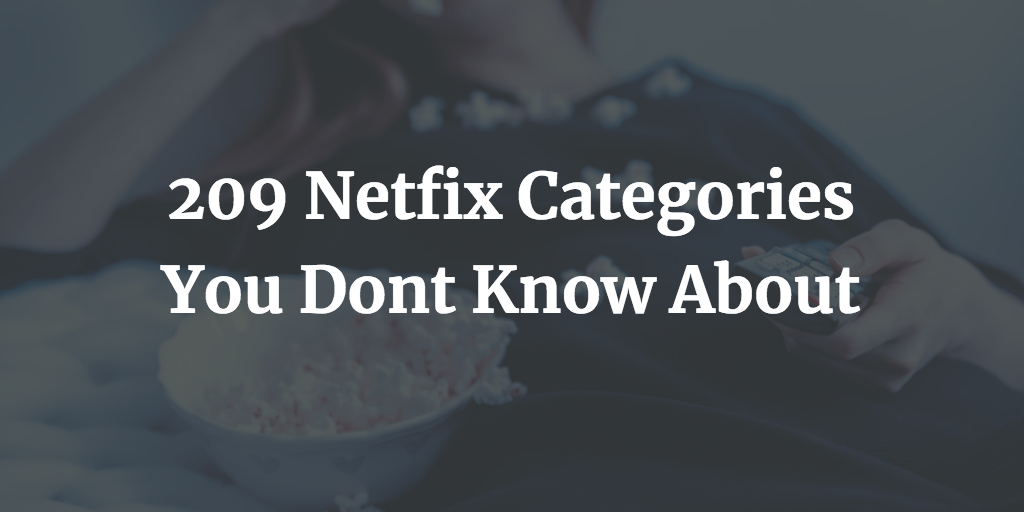
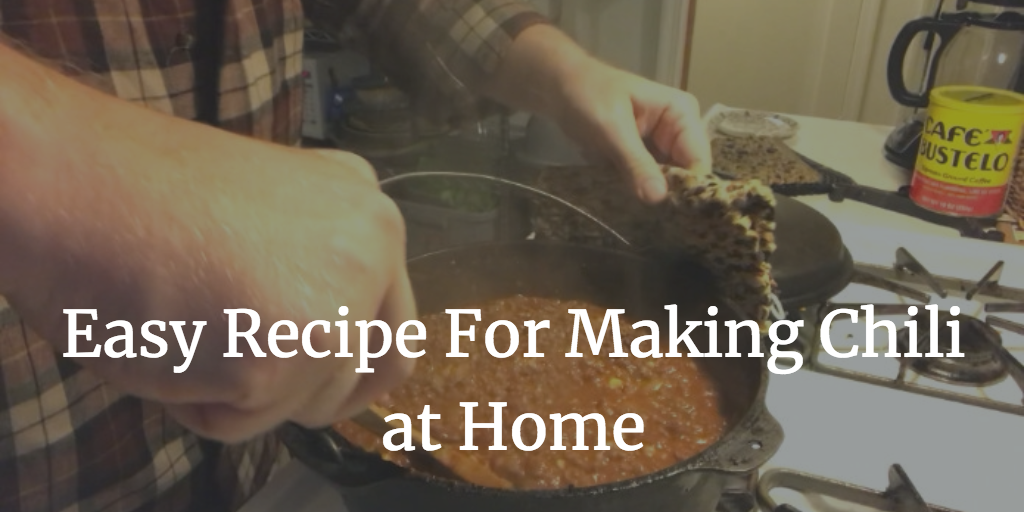
Media Appearances
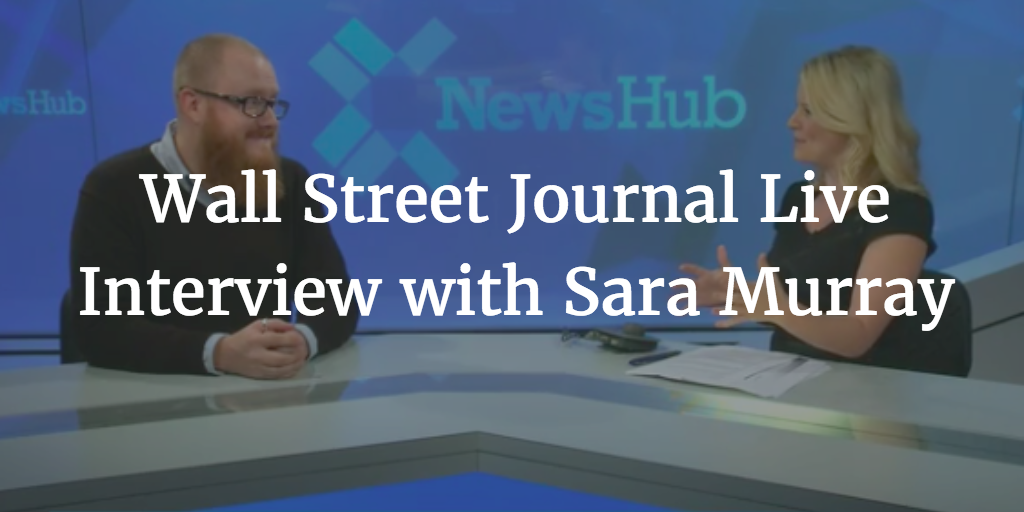
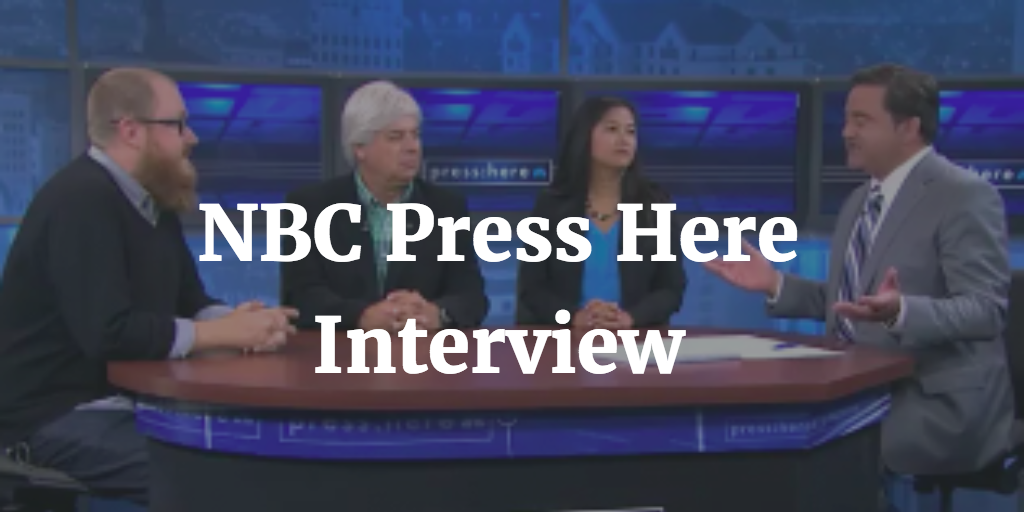
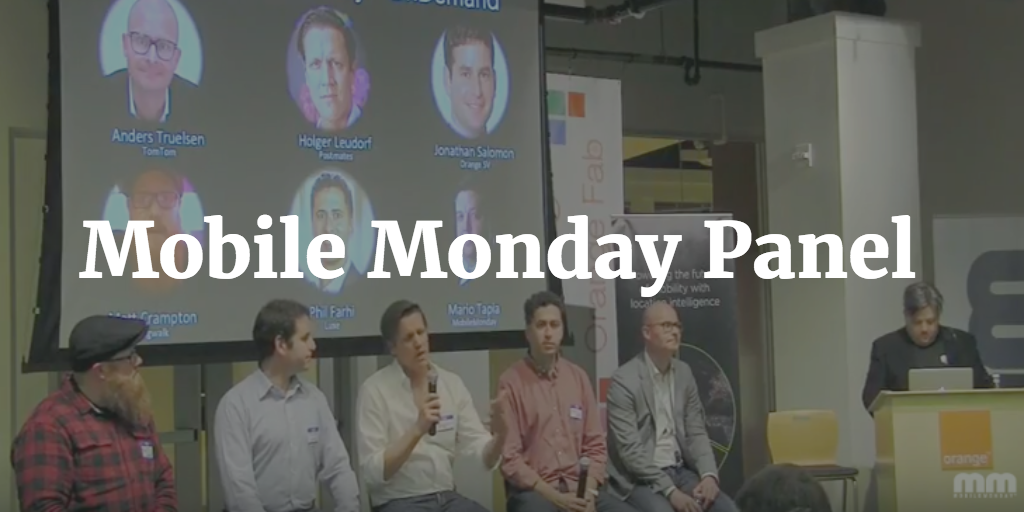
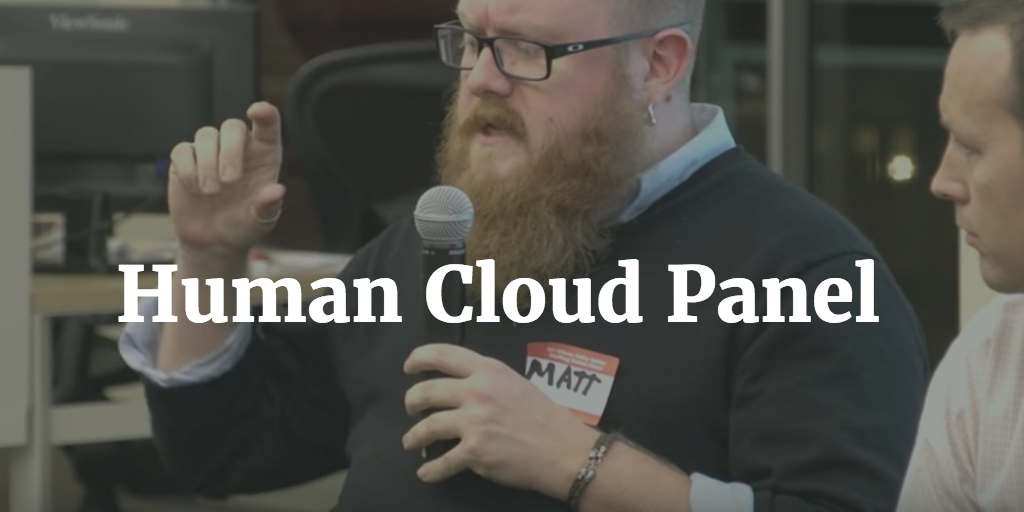
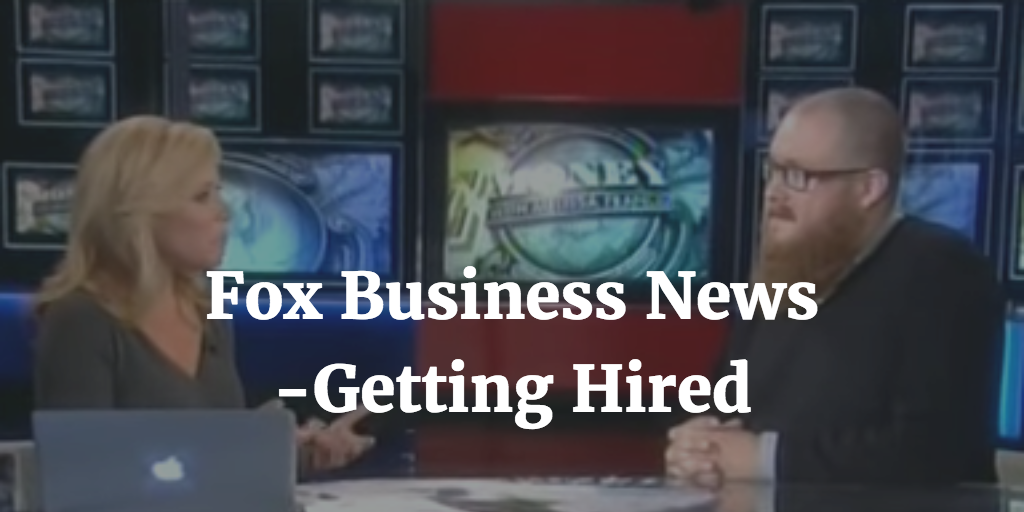
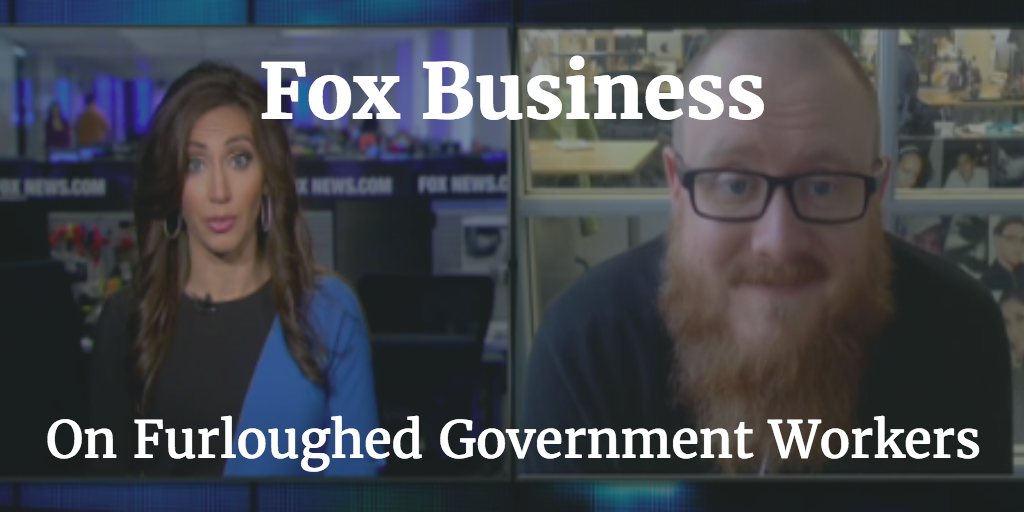
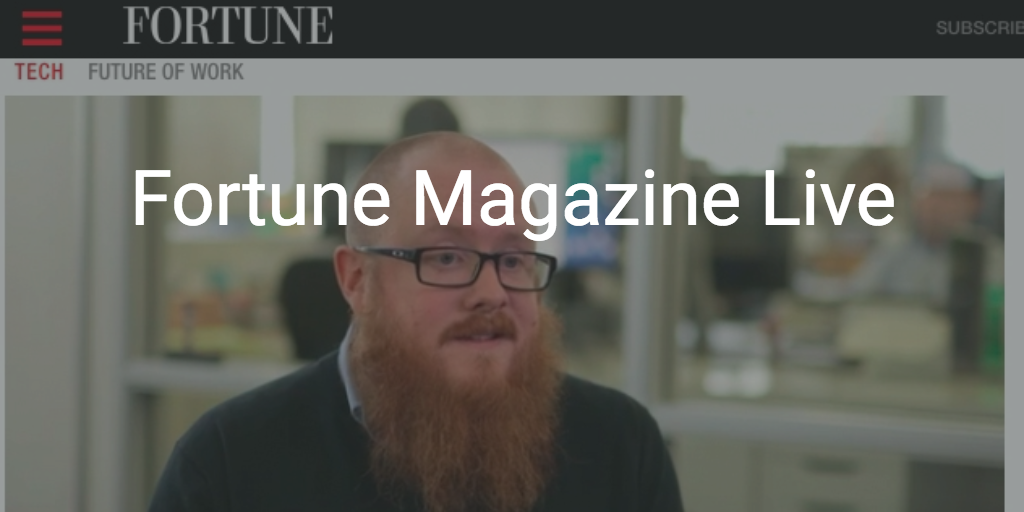
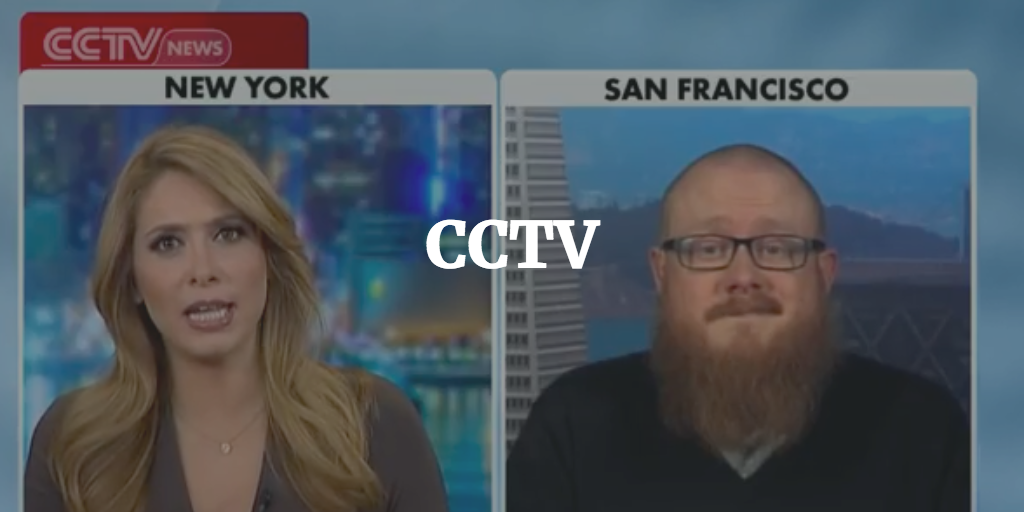
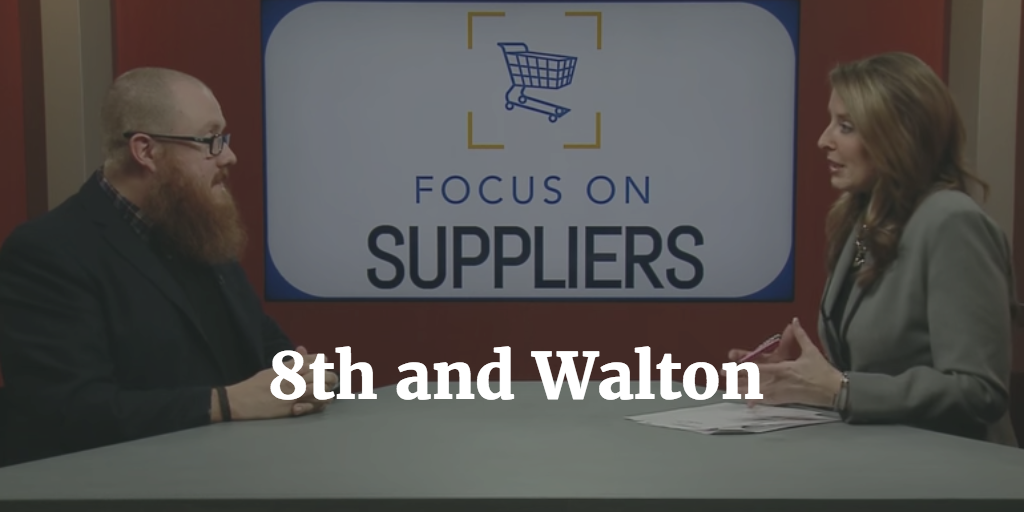
Print Interviews

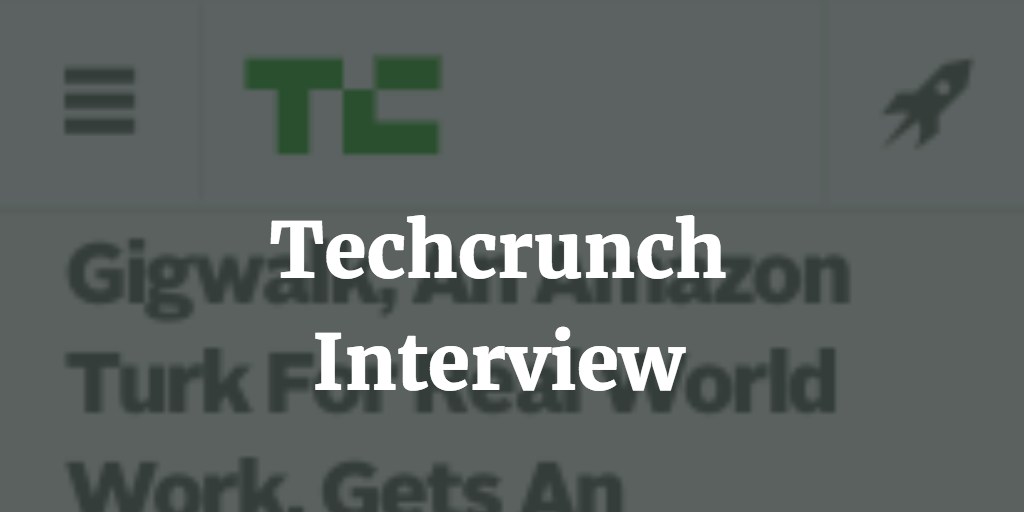
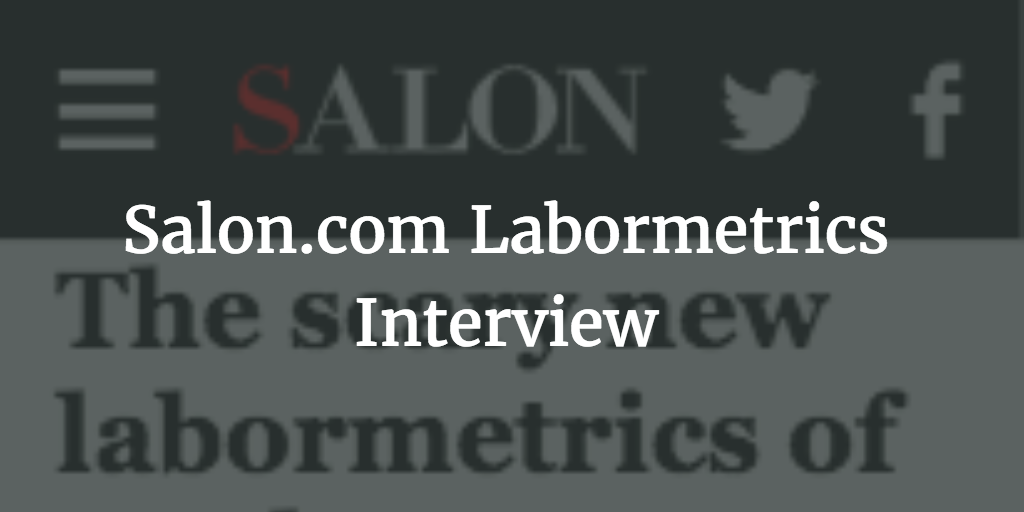
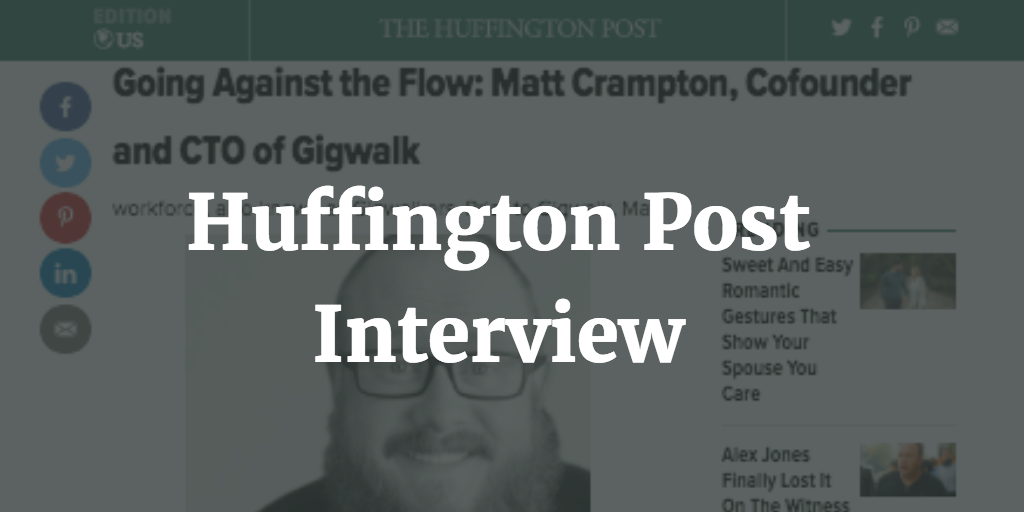
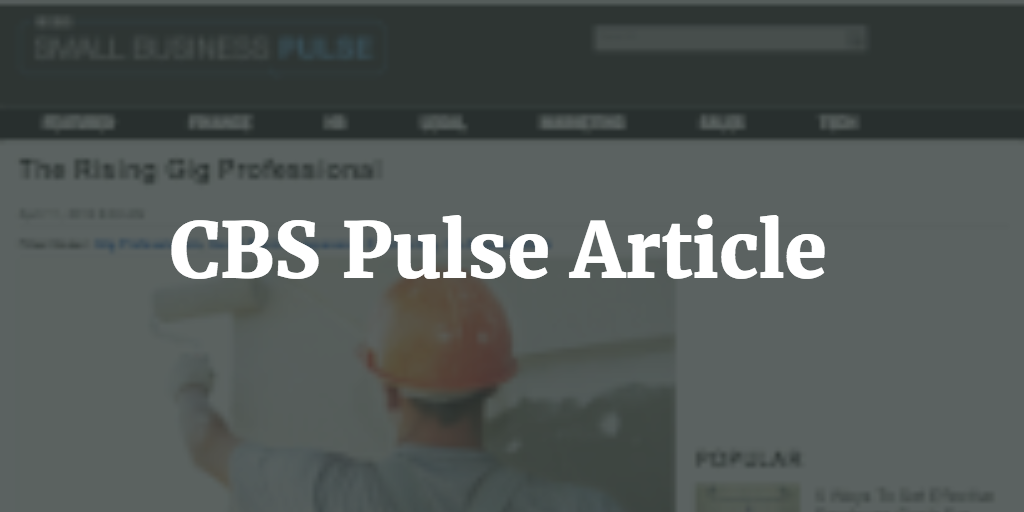
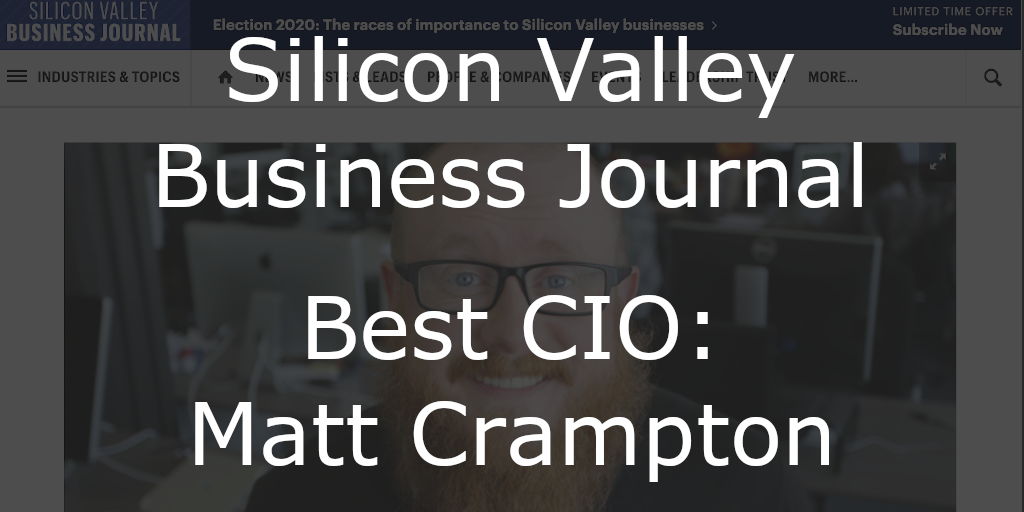